C Exercises: Generate a random permutation of array elements
C Array: Exercise-77 with Solution
Write a program in C to generate random permutations of array elements.
Visual Presentation:
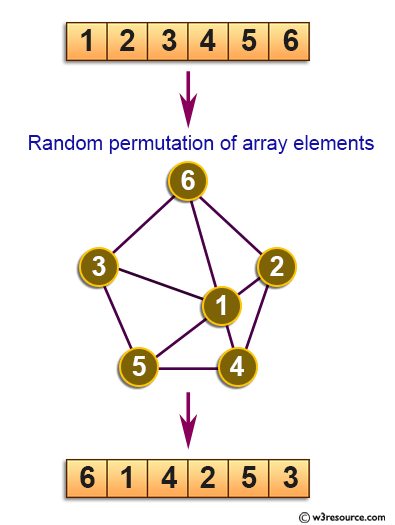
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
// Function to swap the values of two integers
void changeValues(int *a, int *b) {
int temp = *a;
*a = *b;
*b = temp;
}
// Function to display elements of an array
void ArrayDisplay(int arr1[], int n) {
printf("The shuffled elements in the array are: \n");
for (int i = 0; i < n; i++)
printf("%d ", arr1[i]);
printf("\n");
}
// Function to shuffle an array randomly
void shuffleRandom(int arr1[], int n) {
srand(time(NULL)); // Seed for random number generation
for (int i = n - 1; i > 0; i--) {
int j = rand() % (i + 1); // Generate a random index between 0 and i
changeValues(&arr1[i], &arr1[j]); // Swap elements at indices i and j
}
}
int main() {
int arr1[] = {1, 2, 3, 4, 5, 6, 7, 8};
int n = sizeof(arr1) / sizeof(arr1[0]);
int i;
// Print the original array
printf("The given array is: \n");
for (i = 0; i < n; i++) {
printf("%d ", arr1[i]);
}
printf("\n");
shuffleRandom(arr1, n); // Shuffle the array
ArrayDisplay(arr1, n); // Display the shuffled array
return 0;
}
Sample Output:
The given array is: 1 2 3 4 5 6 7 8 The shuffled elements in the array are: 2 8 7 3 4 5 1 6
Flowchart:
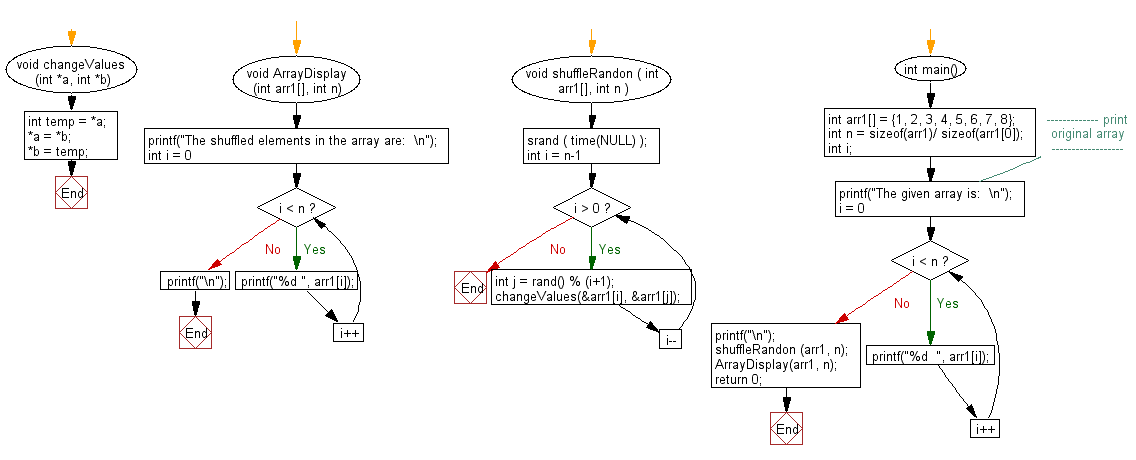
C Programming Code Editor:
Previous: Write a program in C to find largest number possible from the set of given numbers.
Next: Write a program in C to find four array elements whose sum is equal to given number.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics