C Exercises: Copy a file in another name
C File Handling : Exercise-11 with Solution
Write a program in C to copy a file to another name.
Assume that the content of the file test.txt is : test line 1 test line 2 test line 3 test line 4
Sample Solution:
C Code:
#include <stdio.h>
#include <stdlib.h>
void main()
{
FILE *fptr1, *fptr2;
char ch, fname1[20], fname2[20];
printf("\n\n Copy a file in another name :\n");
printf("----------------------------------\n");
printf(" Input the source file name : ");
scanf("%s",fname1);
fptr1=fopen(fname1, "r");
if(fptr1==NULL)
{
printf(" File does not found or error in opening.!!");
exit(1);
}
printf(" Input the new file name : ");
scanf("%s",fname2);
fptr2=fopen(fname2, "w");
if(fptr2==NULL)
{
printf(" File does not found or error in opening.!!");
fclose(fptr1);
exit(2);
}
while(1)
{
ch=fgetc(fptr1);
if(ch==EOF)
{
break;
}
else
{
fputc(ch, fptr2);
}
}
printf(" The file %s copied successfully in the file %s. \n\n",fname1,fname2);
fclose(fptr1);
fclose(fptr2);
getchar();
}
Sample Output:
Copy a file in another name : ---------------------------------- Input the source file name : test.txt Input the new file name : test1.txt The file test.txt copied successfully in the file test1.txt.
Flowchart:
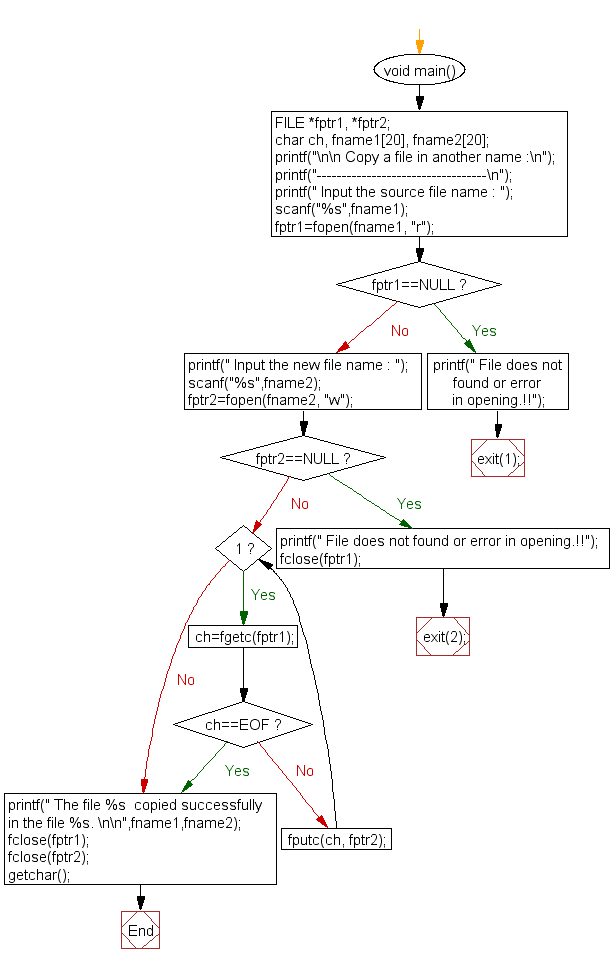
C Programming Code Editor:
Previous: Write a program in C to append multiple lines at the end of a text file.
Next: Write a program in C to merge two files and write it in a new file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics