C Exercises: Swap every two adjacent nodes of a singly linked list
C Singly Linked List : Exercise-40 with Solution
Write a C program to swap every two adjacent nodes of a given singly linked list.
Sample Solution:
C Code:
#include<stdio.h>
#include <stdlib.h>
// Definition for singly-linked list
struct Node {
int data;
struct Node* next;
};
// Function to swap pairs of nodes in a linked list
struct Node* swap_pairs(struct Node* head) {
// If the list is empty or has only one node, no swapping required
if (!head || !head->next) return head;
struct Node *prev = NULL, *curr = head, *next = head->next;
head = next; // Update head to the new starting node after swapping
// Traverse through the list and swap pairs of nodes
while (next) {
curr->next = next->next; // Adjust pointers for swapping
next->next = curr;
// Connect the swapped pair with the previous node or the head if it's the first pair
if (prev) prev->next = next;
prev = curr; // Move to the next pair to be swapped
curr = curr->next;
// Update 'next' for the next pair of nodes to swap
if (curr) next = curr->next;
}
return head; // Return the modified head of the list
}
// Function to create a new node in the linked list
struct Node* newNode(int data) {
struct Node* node = (struct Node*) malloc(sizeof(struct Node)); // Allocate memory for a new node
node->data = data; // Assign data to the new node
node->next = NULL; // Initialize the next pointer as NULL
return node; // Return the newly created node
}
// Function to print the linked list
void printList(struct Node* node) {
while (node != NULL) {
printf("%d ", node->data); // Print the data of the current node
node = node->next; // Move to the next node
}
}
// Main function to demonstrate swapping pairs in a linked list
int main() {
// Create a sample linked list
struct Node* head = newNode(1);
head->next = newNode(2);
head->next->next = newNode(3);
head->next->next->next = newNode(4);
head->next->next->next->next = newNode(5);
printf("Original List:\n");
printList(head); // Display the original linked list
head = swap_pairs(head); // Swap pairs of nodes in the list
printf("\n\nUpdated List after swapping every two adjacent nodes:\n");
printList(head); // Display the modified linked list
return 0;
}
Sample Output:
Original List: 1 2 3 4 5 Updated List after swapping every two adjacent nodes: 2 1 4 3 5
Flowchart :
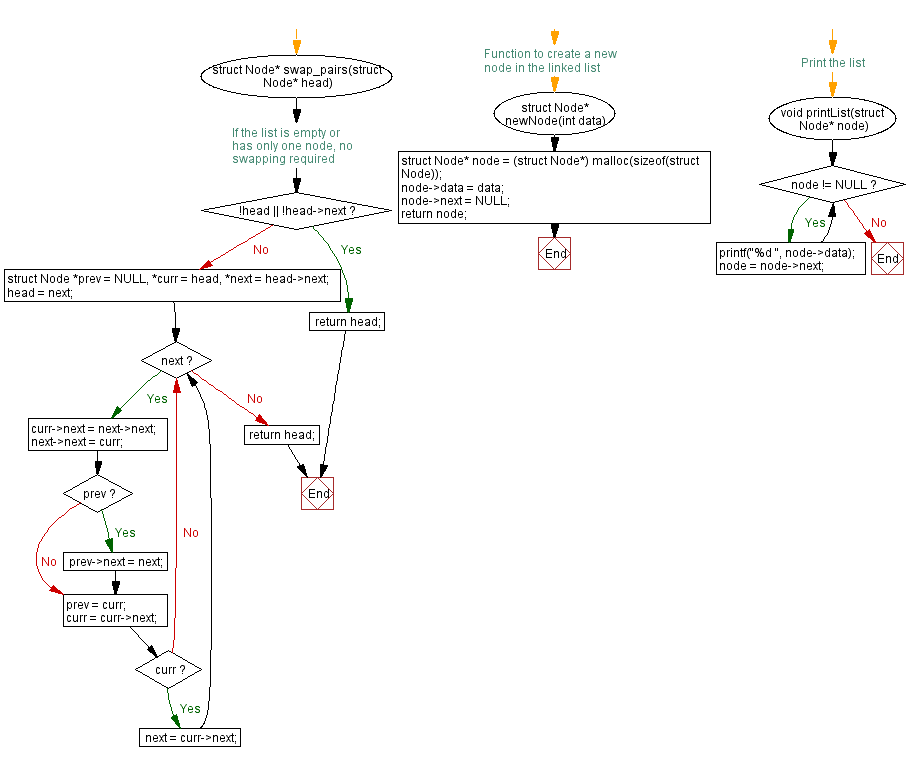
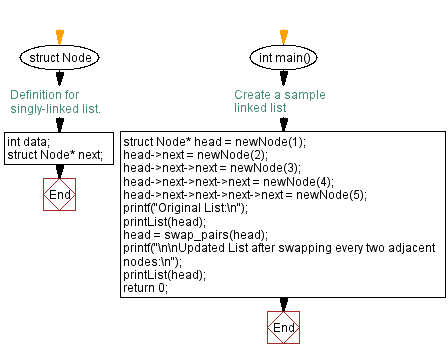
C Programming Code Editor:
Previous: Interleave elements of two linked lists alternatively.
Next: Reverse alternate k nodes of a singly linked list.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics