C#: Check the length of a given string is odd or even
Odd or Even Length of String
Write a C# Sharp program to check the length of a given string is odd or even. Return 'Odd length' if the string length is odd otherwise 'Even length'.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
namespace exercises
{
class Program
{
// Main method where the program execution begins
static void Main(string[] args)
{
// Display original strings and check if their lengths are even or odd
Console.WriteLine("Original string: PHP");
Console.WriteLine("Length of the string: " + test("PHP"));
Console.WriteLine("Original string: javascript");
Console.WriteLine("Length of the string: " + test("javascript"));
Console.WriteLine("Original string: python");
Console.WriteLine("Length of the string: " + test("python"));
}
// Function to determine if the length of a string is even or odd
public static string test(string word)
{
int length = word.Length; // Get the length of the input string
// Check if the length is even or odd using the modulus operator (%)
if (length % 2 == 0) // If the remainder is 0, the length is even
{
return "Even length";
}
else // If the remainder is not 0, the length is odd
{
return "Odd length";
}
}
}
}
Sample Output:
Original string: PHP Convert the letters of the said string into alphabetical order: Odd length Original string: javascript Convert the letters of the said string into alphabetical order: Even length Original string: python Convert the letters of the said string into alphabetical order: Even length
Flowchart:
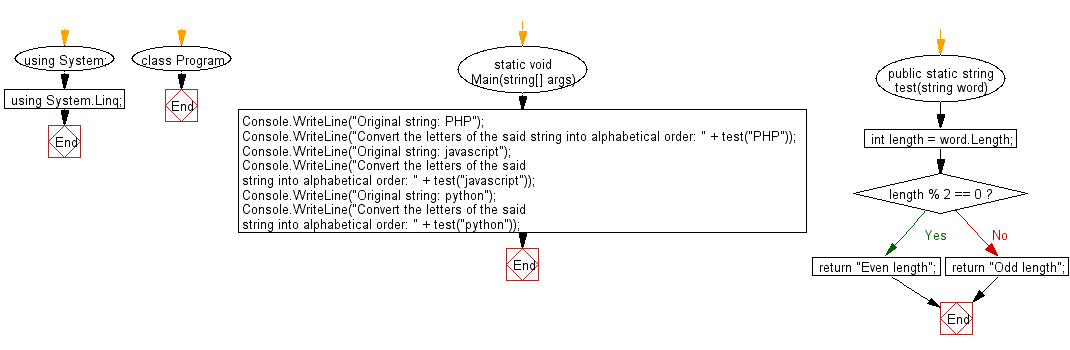
For more Practice: Solve these Related Problems:
- Write a C# program to return "Prime length" if the string length is a prime number.
- Write a C# program to check if the length of the string is divisible by 3 or 5.
- Write a C# program to return the difference between the number of vowels and string length.
- Write a C# program to return the product of string length and the number of uppercase letters.
Go to:
PREV : Alphabetical Order of String Letters.
NEXT : Nth Odd Number.
C# Sharp Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.