C#: Compares two dates
C# Sharp DateTime: Exercise-16 with Solution
Write a C# Sharp program that compares two dates.
Sample Solution:-
C# Sharp Code:
using System;
public class Example16
{
// Main method, entry point of the program
public static void Main()
{
// Define two DateTime instances
DateTime date1 = new DateTime(2016, 8, 1, 0, 0, 0); // August 1, 2016, 00:00:00
DateTime date2 = new DateTime(2016, 8, 1, 12, 0, 0); // August 1, 2016, 12:00:00
// Compare the two DateTime instances
int result = DateTime.Compare(date1, date2);
string relationship; // Define a string to describe the relationship between the two dates
// Determine the relationship based on the comparison result
if (result < 0)
relationship = "is earlier than";
else if (result == 0)
relationship = "is the same time as";
else
relationship = "is later than";
// Display the relationship between the two dates
Console.WriteLine("{0} {1} {2}", date1, relationship, date2);
}
}
Sample Output:
8/1/2016 12:00:00 AM is earlier than 8/1/2016 12:00:00 PM
Flowchart:
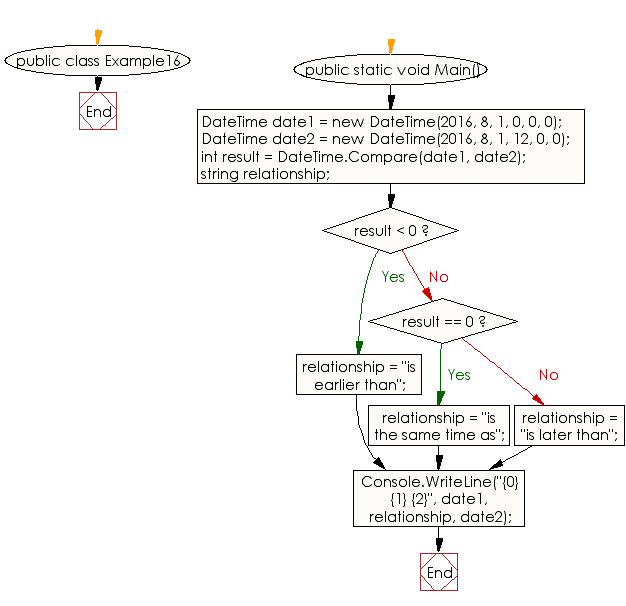
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to display the date of past and future fifteen years of a specified date.
Next: Write a C# Sharp program to create a date one year previously and the date one year in the future compare to the current date.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics