C#: Convert the value of the current DateTime object to its equivalent short time string representation
C# Sharp DateTime : Exercise-35 with Solution
Write a C# Sharp program to convert the current DateTime object value to its equivalent short time string representation.
Sample Solution:-
C# Sharp Code:
using System;
using System.Threading;
using System.Globalization;
class Example35
{
public static void Main()
{
// Message explaining the source of date and time patterns
string msg1 = "The date and time patterns are defined in the DateTimeFormatInfo \n" +
"object associated with the current thread culture.\n";
// Initialize a DateTime object.
Console.WriteLine("Initialize the DateTime object to August 16, 2016 3:02:15 AM.\n");
DateTime myDateTime = new System.DateTime(2016, 8, 16, 3, 2, 15);
// Display information about the source of date and time patterns.
Console.WriteLine(msg1);
// Display the name of the current culture.
CultureInfo ci = Thread.CurrentThread.CurrentCulture;
Console.WriteLine("Current culture: \"{0}\"\n", ci.Name);
// Display the long date pattern and its associated string.
Console.WriteLine("Long date pattern: \"{0}\"", ci.DateTimeFormat.LongDatePattern);
Console.WriteLine("Long date string: \"{0}\"\n", myDateTime.ToLongDateString());
// Display the long time pattern and its associated string.
Console.WriteLine("Long time pattern: \"{0}\"", ci.DateTimeFormat.LongTimePattern);
Console.WriteLine("Long time string: \"{0}\"\n", myDateTime.ToLongTimeString());
// Display the short date pattern and its associated string.
Console.WriteLine("Short date pattern: \"{0}\"", ci.DateTimeFormat.ShortDatePattern);
Console.WriteLine("Short date string: \"{0}\"\n", myDateTime.ToShortDateString());
// Display the short time pattern and its associated string.
Console.WriteLine("Short time pattern: \"{0}\"", ci.DateTimeFormat.ShortTimePattern);
Console.WriteLine("Short time string: \"{0}\"\n", myDateTime.ToShortTimeString());
}
}
Sample Output:
Initialize the DateTime object to August 16, 2016 3:02:15 AM. The date and time patterns are defined in the DateTimeFormatInfo object associated with the current thread culture. Current culture: "en-US" Long date pattern: "dddd, MMMM d, yyyy" Long date string: "Tuesday, August 16, 2016" Long time pattern: "h:mm:ss tt" Long time string: "3:02:15 AM" Short date pattern: "M/d/yyyy" Short date string: "8/16/2016" Short time pattern: "h:mm tt" Short time string: "3:02 AM"
Flowchart:
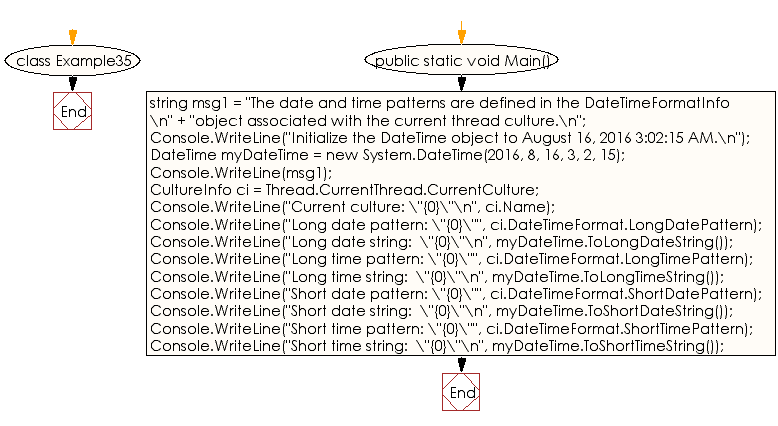
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to convert the value of the current DateTime object to its equivalent short date string representation.
Next: Write a C# Sharp program to convert the value of the current DateTime object to its equivalent string representation using the formatting conventions of the current culture.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics