C#: Check whether the given year, month and day are the current or not
C# Sharp DateTime: Exercise-43 with Solution
Write a program in C# Sharp to check whether the given year, month and day are current or not.
Sample Solution:-
C# Sharp Code:
using System;
class dttimeex43
{
static void Main()
{
int yr, mn, dt;
// Displaying the purpose of the program to the user.
Console.Write("\n\n Check whether the given year, month, and day is the current or not :\n");
Console.Write("------------------------------------------------------------------------\n");
// Input day, month, and year from the user.
Console.Write(" Input the Day : ");
dt = Convert.ToInt32(Console.ReadLine());
Console.Write(" Input the Month : ");
mn = Convert.ToInt32(Console.ReadLine());
Console.Write(" Input the Year : ");
yr = Convert.ToInt32(Console.ReadLine());
// Create a DateTime object using the provided day, month, and year.
DateTime value = new DateTime(yr, mn, dt);
// Display the formatted date to the user in the "dd/MM/yyyy" format.
Console.WriteLine(" The formatted Date is : {0}", value.ToString("dd/MM/yyyy"));
// Check if the provided date is the current date.
Console.WriteLine(" The current date status : {0}\n", value == DateTime.Today);
}
}
Sample Output:
Check whether the given year, month and day is the current or not : ------------------------------------------------------------------------ Input the Day : 12 Input the Month : 06 Input the Year : 2017 The formatted Date is : 12/06/2017 The current date status : True
Flowchart:
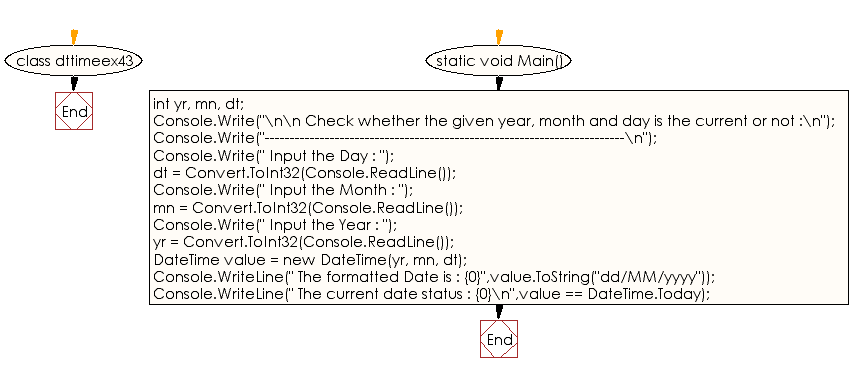
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to convert the specified string representation of a date and time to its DateTime equivalent using the specified format, culture-specific format information, and style.
Next: Write a program in C# Sharp to compute what day was yesterday.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics