C#: Calculate the size of file
C# Sharp LINQ : Exercise-16 with Solution
Write a program in C# Sharp to calculate size of file using LINQ
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
using System.IO;
// Define a class named LinqExercise16
class LinqExercise16
{
// Main method, the entry point of the program
static void Main(string[] args)
{
// Get an array of file names present in the directory "/home/w3r/abcd/"
string[] dirfiles = Directory.GetFiles("/home/w3r/abcd/");
// Note: There are three files in the directory "abcd" named abcd.txt, simple_file.txt, and xyz.txt
// Display information about the program
Console.Write("\nLINQ : Calculate the size of file : ");
Console.Write("\n------------------------------------\n");
// Calculate the average file size using LINQ
var avgFsize = dirfiles.Select(file => new FileInfo(file).Length).Average();
// Convert the average file size from bytes to megabytes and round to one decimal place
avgFsize = Math.Round(avgFsize / 10, 1);
// Display the average file size in megabytes
Console.WriteLine("The Average file size is {0} MB", avgFsize);
Console.ReadLine(); // Wait for user input before closing the program
}
}
Sample Output:
The Average file size is 3.4 MB
Flowchart:
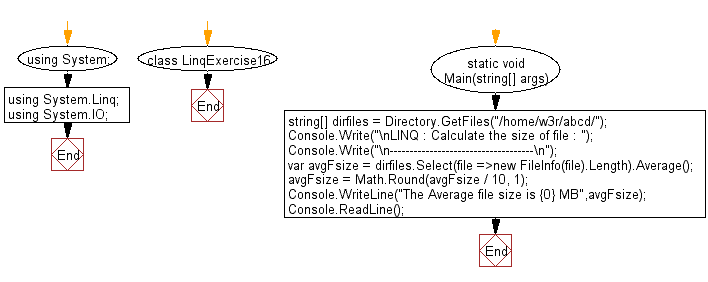
C# Sharp Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Program to Count File Extensions and Group it using LINQ.
Next: Write a program in C# Sharp to Remove Items from List using remove function by passing object.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics