C#: Using multiple WHERE clause to find the positive numbers within the list
C# Sharp LINQ : Exercise-2 with Solution
Write a program in C# Sharp to find the positive numbers from a list of numbers using two where conditions in LINQ Query.
Sample Solution:
C# Sharp Code:
using System;
using System.Linq;
class LinqExercise2
{
static void Main()
{
// Define an integer array
int[] n1 = {
1, 3, -2, -4, -7, -3, -8, 12, 19, 6, 9, 10, 14
};
// Display a message indicating the purpose of the LINQ operation
Console.Write("\nLINQ : Using multiple WHERE clause to find the positive numbers within the list : ");
Console.Write("\n-----------------------------------------------------------------------------");
// Define a LINQ query using multiple 'where' clauses to filter numbers within a specific range
var nQuery =
from VrNum in n1
where VrNum > 0 // Filter numbers greater than 0
where VrNum < 12 // Further filter numbers less than 12
select VrNum;
// Display the selected numbers that satisfy the conditions
Console.Write("\nThe numbers within the range of 1 to 11 are : \n");
foreach(var VrNum in nQuery)
{
Console.Write("{0} ", VrNum);
}
Console.Write("\n\n"); // Add newline for formatting
}
}
Sample Output:
LINQ : Using multiple WHERE clause to find the positive numbers within the list : ----------------------------------------------------------------------------- The numbers within the range of 1 to 11 are : 1 3 6 9 10
Flowchart:
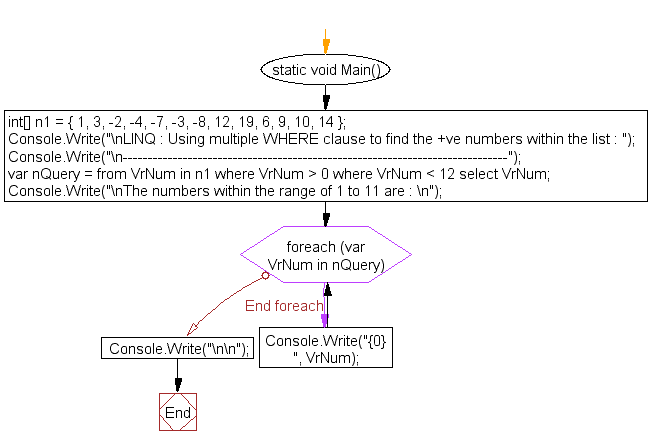
C# Sharp Practice online:
Contribute your code and comments through Disqus.
Previous: Write a program in C# Sharp to shows how the three parts of a query operation execute.
Next: Write a program in C# Sharp to find the number of an array and the square of each number which is more than 20.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics