C#: Compare two strings in different ways produce three different results
C# Sharp String: Exercise-28 with Solution
Write a C# Sharp program to compare two strings in following three different ways produce three different results.
a. using linguistic comparison for the en-US culture.
b. using linguistic case-sensitive comparison for the en-US culture.
c. using an ordinal comparison. It illustrates how the three methods of comparison.
Sample Solution:-
C# Sharp Code:
using System;
using System.Globalization;
public class Example28
{
public static void Main()
{
// Declare and initialize two strings for comparison
string str1 = "sister";
string str2 = "Sister";
// Declare a variable to hold the relationship between the strings
string relation;
int result;
// Cultural (linguistic) comparison.
result = String.Compare(str1, str2, new CultureInfo("en-US"),
CompareOptions.None);
// Determine the relationship between str1 and str2 based on the comparison result
if (result > 0)
relation = "comes after";
else if (result == 0)
relation = "is the same as";
else
relation = "comes before";
// Display the relationship between str1 and str2 for cultural comparison
Console.WriteLine("'{0}' {1} '{2}'.",
str1, relation, str2);
// Cultural (linguistic) case-insensitive comparison.
result = String.Compare(str1, str2, new CultureInfo("en-US"),
CompareOptions.IgnoreCase);
// Determine the relationship between str1 and str2 for case-insensitive comparison
if (result > 0)
relation = "comes after";
else if (result == 0)
relation = "is the same as";
else
relation = "comes before";
// Display the relationship between str1 and str2 for case-insensitive comparison
Console.WriteLine("'{0}' {1} '{2}'.",
str1, relation, str2);
// Culture-insensitive ordinal comparison.
result = String.CompareOrdinal(str1, str2);
// Determine the relationship between str1 and str2 for ordinal comparison
if (result > 0)
relation = "comes after";
else if (result == 0)
relation = "is the same as";
else
relation = "comes before";
// Display the relationship between str1 and str2 for ordinal comparison
Console.WriteLine("'{0}' {1} '{2}'.",
str1, relation, str2);
}
}
Sample Output:
'sister' comes before 'Sister'. 'sister' is the same as 'Sister'. 'sister' comes after 'Sister'.
Flowchart:
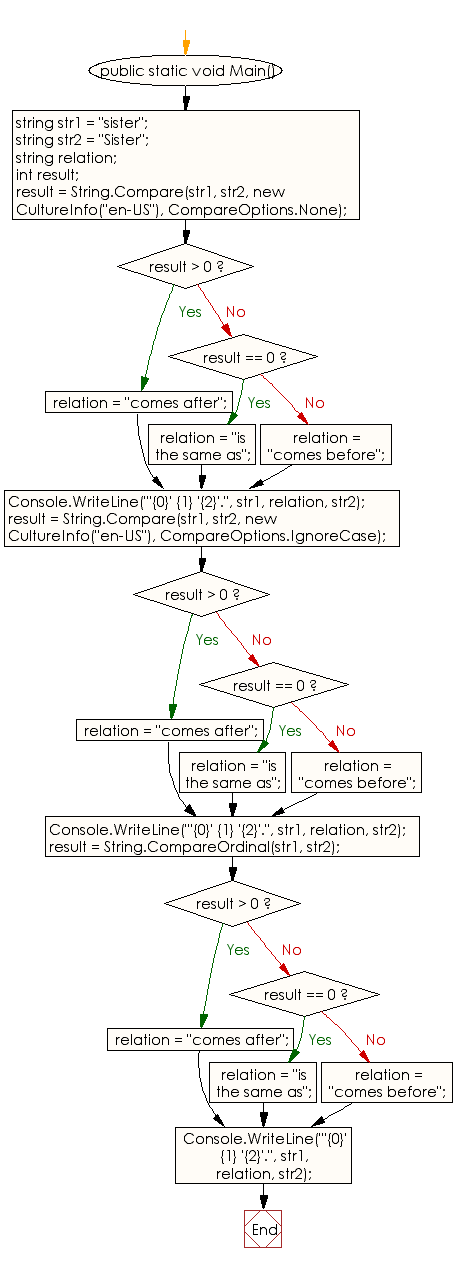
C# Sharp Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a C# Sharp program to demonstrate how culture can affect a comparison.
Next: Write a C# Sharp program to compare three versions of the letter "I". The results are affected by the choice of culture, whether case is ignored, and whether an ordinal comparison is performed.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics