Java: Print the area and perimeter of a rectangle
Java Basic: Exercise-13 with Solution
Write a Java program to print the area and perimeter of a rectangle.
Java: Perimeter of a rectangle
A perimeter is a path that surrounds a two-dimensional shape. The word comes from the Greek peri (around) and meter (measure). The perimeter can be used to calculate the length of fence required to surround a yard or garden. Following image represents the perimeter of a rectangle.
Java: Area of a rectangle
In Euclidean plane geometry, a rectangle is a quadrilateral with four right angles. To find the area of a rectangle, multiply the length by the width.
A rectangle with four sides of equal length is a square.
Following image represents the area of a rectangle.
Pictorial Presentation:
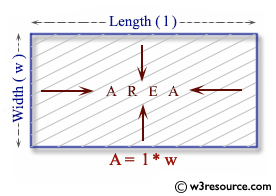
Sample Solution:
Java Code:
public class Exercise13 {
public static void main(String[] strings) {
// Define constants for the width and height of the rectangle
final double width = 5.6;
final double height = 8.5;
// Calculate the perimeter of the rectangle
double perimeter = 2 * (height + width);
// Calculate the area of the rectangle
double area = width * height;
// Print the calculated perimeter using placeholders for values
System.out.printf("Perimeter is 2*(%.1f + %.1f) = %.2f \n", height, width, perimeter);
// Print the calculated area using placeholders for values
System.out.printf("Area is %.1f * %.1f = %.2f \n", width, height, area);
}
}
Explanation:
In the exercise above -
- Define constant values for the rectangle's width (5.6) and height (8.5).
- Calculate the rectangle perimeter using the formula: 2 * (height + width).
- Calculate the rectangle area using the formula: width * height.
- Prints the perimeter and area values to the console using printf statements with formatted output to display the results with specific decimal precision.
Sample Output:
Perimeter is 2*(8.5 + 5.6) = 28.20 Area is 5.6 * 8.5 = 47.60
Flowchart:
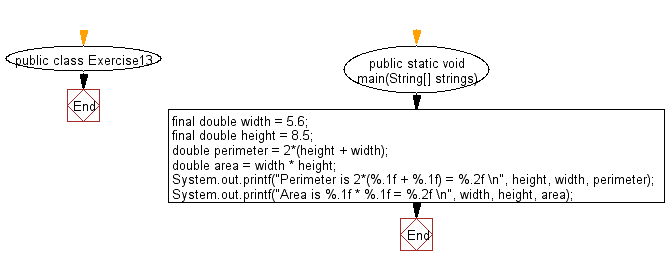
Java Code Editor:
Previous: Write a Java program that takes five numbers as input to calculate and print the average of the numbers.
Next: Write a Java program to print an American flag on the screen.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics