Java: Print mode values from a given a sequence of integers
Java Basic: Exercise-226 with Solution
Write a Java program to print mode values from a given sequence of integers. The mode value is the element that occurs most frequently. If there are several mode values, print them in ascending order.
Input:
A sequence of integer’s ai (1 ≤ ai ≤ 100). The number of integers is less than or equals to 100.
Visual Presentation:
Sample Solution:
Java Code:
// Importing the Scanner class for user input
import java.util.Scanner;
// Main class named "Main"
public class Main {
// Main method to execute the program
public static void main(String[] args) {
// Creating a Scanner object for user input
Scanner input = new Scanner(System.in);
// Array to store the count of occurrences for each integer (0-99)
int cnt[] = new int[100];
// Variable to track the current index in the loop
int i;
// Prompting the user to input the number of integers
System.out.println("How many integers would you like to input (Max. 100)?");
// Reading the input value for the number of integers
int x = input.nextInt();
// Prompting the user to input the integers
System.out.println("Input the integers:");
// Loop to process user input and update the count array
for (i = 0; i < x; i++) {
// Reading the next integer from the input
int n = input.nextInt();
// Updating the count array based on the input integer
cnt[--n]++;
}
// Variable to store the maximum count
int max = 0;
// Loop to find the maximum count in the count array
for (int n : cnt) {
if (max < n) {
max = n;
}
}
// Prompting the user with the mode value(s) in ascending order
System.out.println("Mode value(s) in ascending order:");
// Loop to find and print the mode value(s)
for (i = 0; i < cnt.length; i++) {
if (cnt[i] == max) {
// Printing the mode value (adding 1 to get the original value)
System.out.println(i + 1);
}
}
}
}
Sample Output:
How many integers would you like to input(Max.100?) 5 Input the integers: 25 35 15 5 45 Mode value(s)in ascending order: 5 15 25 35 45
Flowchart:
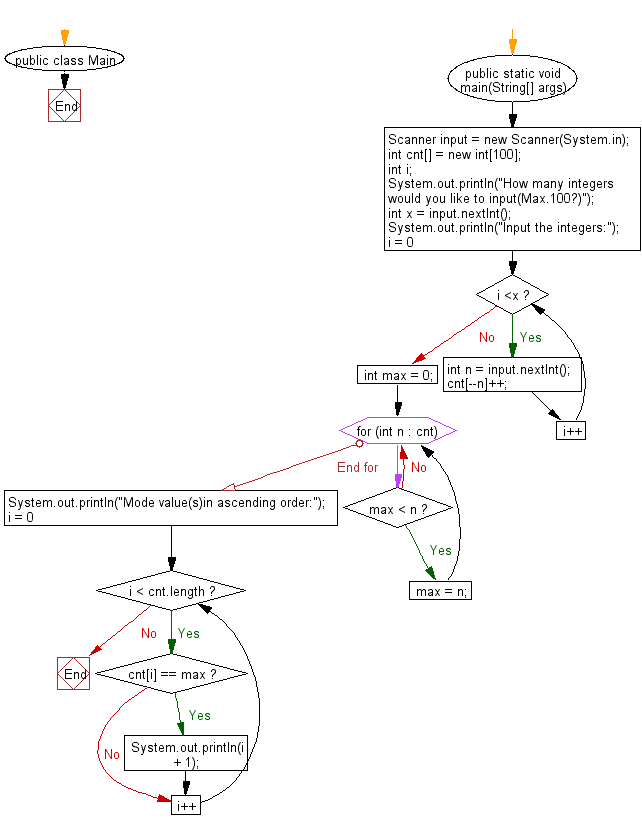
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Write a Java program to that reads a date (from 2004/1/1 to 2004/12/31) and prints the day of the date. Jan. 1, 2004, is Thursday. Note that 2004 is a leap year.
Next: Write a Java program which reads a text (only alphabetical characters and spaces.) and prints two words. The first one is the word which is arise most frequently in the text. The second one is the word which has the maximum number of letters.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics