Java: Add all the elements of a specified tree set to another tree set
Java Collection, TreeSet Exercises: Exercise-3 with Solution
Write a Java program to add all the elements of a specified tree set to another tree set.
Sample Solution:
Java Code:
import java.util.TreeSet;
public class Exercise3 {
public static void main(String[] args) {
TreeSet<String> tree_set1 = new TreeSet<String>();
tree_set1.add("Red");
tree_set1.add("Green");
tree_set1.add("Orange");
System.out.println("Tree set1: "+tree_set1);
TreeSet<String> tree_set2 = new TreeSet<String>();
tree_set2.add("Pink");
tree_set2.add("White");
tree_set2.add("Black");
System.out.println("Tree set2: "+tree_set2);
// adding treetwo to treeone
tree_set1.addAll(tree_set2);
System.out.println("Tree set1: "+tree_set1);
}
}
Sample Output:
Tree set1: [Green, Orange, Red] Tree set2: [Black, Pink, White] Tree set1: [Black, Green, Orange, Pink, Red, White]
Flowchart:
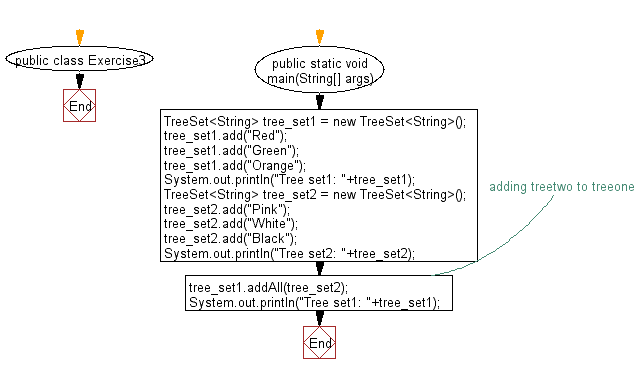
Java Code Editor:
Contribute your code and comments through Disqus.
Previous: Iterate through all elements in a tree set.
Next: Create a reverse order view of the elements contained in a given tree set.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics