Java Program: String input validation for vowels
Java Exception Handling: Exercise-7 with Solution
Write a Java program to create a method that takes a string as input and throws an exception if the string does not contain vowels.
Sample Solution:
Java Code:
public class Vowel_Check {
public static void main(String[] args) {
try {
String text = "Java handling and managing exceptions ";
// String text = "Typy gyps fly.";
System.out.println("Original string: " + text);
checkVowels(text);
System.out.println("String contains vowels.");
} catch (NoVowelsException e) {
System.out.println("Error: " + e.getMessage());
}
}
public static void checkVowels(String text) throws NoVowelsException {
boolean containsVowels = false;
String vowels = "aeiouAEIOU";
for (int i = 0; i < text.length(); i++) {
char ch = text.charAt(i);
if (vowels.contains(String.valueOf(ch))) {
containsVowels = true;
break;
}
}
if (!containsVowels) {
throw new NoVowelsException("String does not contain any vowels.");
}
}
}
class NoVowelsException extends Exception {
public NoVowelsException(String message) {
super(message);
}
}
Sample Output:
Original string: Java handling and managing exceptions String contains vowels.
Original string: Typy gyps fly. Error: String does not contain any vowels
Explanation:
In the above exercise,
- The main method is defined in the Vowel_Check class and serves as the program entry point.
- In the main method, a string of text is initialized with a value. This string represents the input to be checked for vowels.
- The checkVowels method is called, passing the text string as an argument. This method checks if the string contains vowels.
- Inside the checkVowels method, a boolean variable containsVowels is initialized as false. This variable tracks whether vowels are found in the string.
- A for loop iterates through each character of the text string.
- After the loop, if containsVowels is still false, it means no vowels were found in the string. In this case, a NoVowelsException is thrown with the message "String does not contain any vowels."
- The NoVowelsException class is a custom exception class that extends the base Exception class. It provides a constructor that takes a message parameter and passes it to the superclass constructor using the super keyword.
- If a NoVowelsException occurs in the main method, it is caught, and an appropriate error message is printed.
Flowchart:
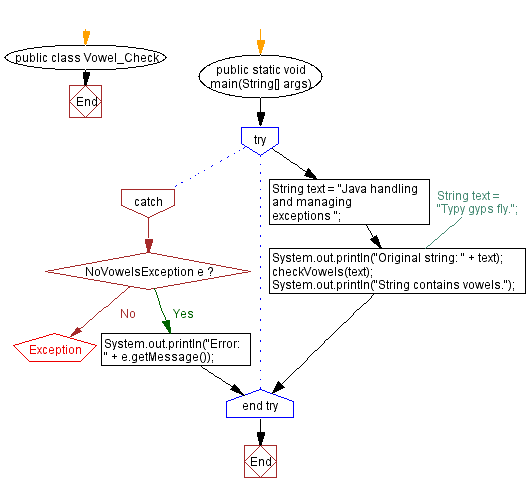
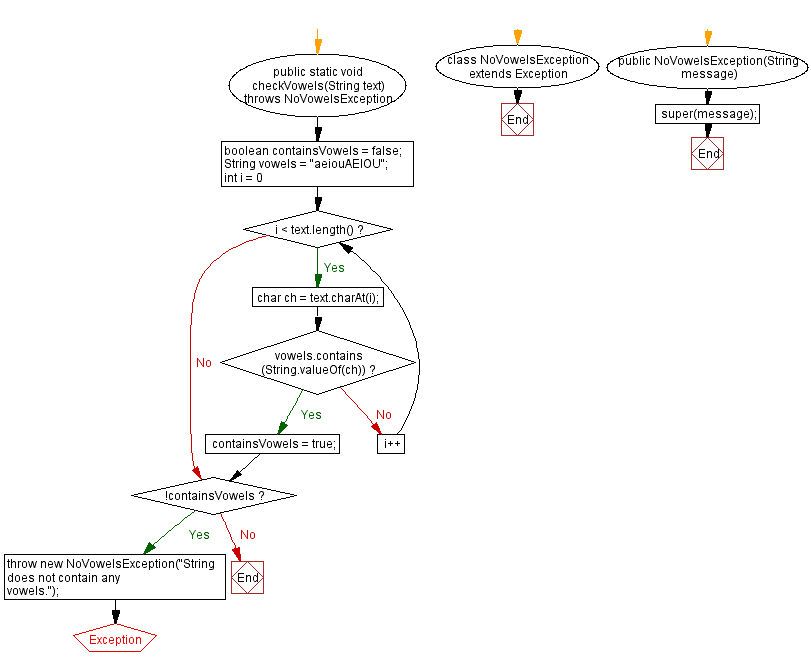
Java Code Editor:
Improve this sample solution and post your code through Disqus
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics