Java Interface: Implement Animal interface with a method bark()
Java Interface: Exercise-2 with Solution
Write a Java program to create a Animal interface with a method called bark() that takes no arguments and returns void. Create a Dog class that implements Animal and overrides speak() to print "Dog is barking".
Sample Solution:
Java Code:
// Animal.java
// Interface Animal
// Declare the Animal interface
public interface Animal {
// Declare the abstract method "bark" that classes implementing this interface must provide
void bark();
}
// Dog.java
// Class Dog
// Declare the Dog class, which implements the Animal interface
public class Dog implements Animal {
// Implement the "bark" method required by the Animal interface
@Override
public void bark() {
// Print a message indicating that the Dog is barking
System.out.println("Dog is barking!");
}
}
// Main.java
// Class Main
// Declare the Main class
public class Main {
public static void main(String[] args) {
// Create an instance of the Dog class
Dog dog = new Dog();
// Call the "bark" method on the Dog instance
dog.bark();
}
}
Sample Output:
Dog is barking!
Explanation:
This exercise defines an interface called "Animal" with a method bark() that takes no arguments and returns void. We then define a class called "Dog" that implements the "Animal" interface and overrides the bark() method to print "Dog is barking" to the console.
In the Main class we create an instance of the "Dog" class and call the bark() method, which should print 'Dog is barking' to the console.
Flowchart of Interface Animal:
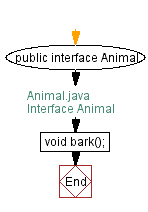
Flowchart of Class Dog:
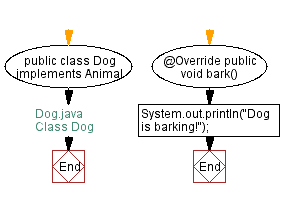
Flowchart of Class Main:

Java Code Editor:
Previous: Shape with the getArea() method, implement the Shape interface.
Next: Implement the Flyable interface.
What is the difficulty level of this exercise?
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics