Java Polymorphism: Vehicle base class with Car and Motorcycle subclasses
Write a Java program to create a base class Vehicle with methods startEngine() and stopEngine(). Create two subclasses Car and Motorcycle. Override the startEngine() and stopEngine() methods in each subclass to start and stop the engines differently.
Sample Solution:
Java Code:
// Vehicle.java
// Define the abstract Vehicle class
abstract class Vehicle {
// Abstract method to start the engine
public abstract void startEngine();
// Abstract method to stop the engine
public abstract void stopEngine();
}
// Car.java
// Define the Car class that extends Vehicle
class Car extends Vehicle {
// Override the startEngine method
@Override
public void startEngine() {
// Print that the car engine started with a key
System.out.println("Car engine started with a key.");
}
// Override the stopEngine method
@Override
public void stopEngine() {
// Print that the car engine stopped when the key was turned off
System.out.println("Car engine stopped when the key was turned off.");
}
}
// Motorcycle.java
// Define the Motorcycle class that extends Vehicle
class Motorcycle extends Vehicle {
// Override the startEngine method
@Override
public void startEngine() {
// Print that the motorcycle engine started with a kick-start
System.out.println("Motorcycle engine started with a kick-start.");
}
// Override the stopEngine method
@Override
public void stopEngine() {
// Print that the motorcycle engine stopped when ignition was turned off
System.out.println("Motorcycle engine stopped when ignition was turned off.");
}
}
// Main.java
// Define the Main class
public class Main {
// Main method, program entry point
public static void main(String[] args) {
// Create a Vehicle reference to a Car object
Vehicle car = new Car();
// Create a Vehicle reference to a Motorcycle object
Vehicle motorcycle = new Motorcycle();
// Start and stop the engine for the car
startAndStopEngine(car);
// Start and stop the engine for the motorcycle
startAndStopEngine(motorcycle);
}
// Method to start and stop the engine of a given vehicle
public static void startAndStopEngine(Vehicle vehicle) {
// Start the engine of the vehicle
vehicle.startEngine();
// Stop the engine of the vehicle
vehicle.stopEngine();
}
}
Output:
Car engine started with a key. Car engine stopped when the key was turned off. Motorcycle engine started with a kick-start. Motorcycle engine stopped when ignition was turned off.
Explanation:
In the above exercise -
- The "Vehicle" class is the base abstract class, and Car and Motorcycle are its concrete subclasses. Each subclass overrides the startEngine() and stopEngine() methods to provide different ways of starting and stopping the engines based on the vehicle type.
- In the Main class, we have a static method startAndStopEngine(Vehicle vehicle) that takes an object of the base class Vehicle as a parameter. Inside this method, we call the startEngine() and stopEngine() methods on the vehicle object. Since the startAndStopEngine method takes a Vehicle type parameter, it can accept Car and Motorcycle objects,
Flowchart:
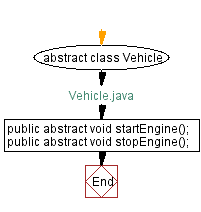
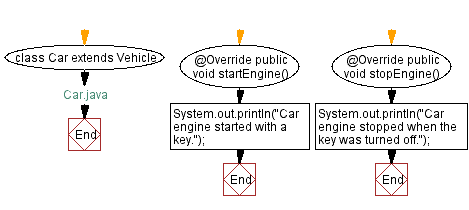
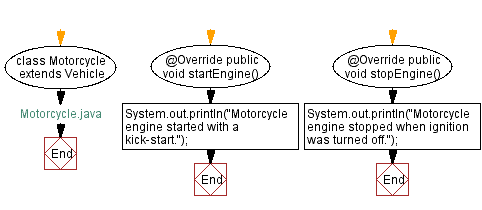
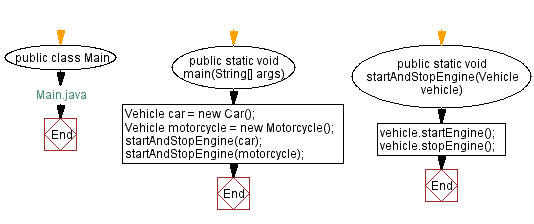
For more Practice: Solve these Related Problems:
- Write a Java program where the "Vehicle" class includes a method to determine fuel efficiency.
- Write a Java program where the "Vehicle" class implements an automatic engine shut-off feature.
- Write a Java program where the "Vehicle" class includes a method to estimate remaining travel distance based on fuel.
- Write a Java program where the "Vehicle" class allows setting different engine modes (eco, sport, etc.).
Go to:
Java Code Editor:
Contribute your code and comments through Disqus.
PREV : Animal base class with Lion, Tiger, and Panther subclasses.
NEXT : Shape base class with Circle and Cylinder subclasses.
What is the difficulty level of this exercise?