Text input and display in JavaFX
JavaFx Basic: Exercise-4 with Solution
Write a JavaFX application with a text input field and a button. When the button is clicked, display the text entered in the input field in a label.
Sample Solution:
JavaFx Code:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.control.TextField;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
// Create a label to display the entered text.
Label resultLabel = new Label("Result will be shown here.");
// Create a text input field and a button.
TextField inputField = new TextField();
Button displayButton = new Button("Display Text");
// Set an action for the button.
displayButton.setOnAction(event -> {
String enteredText = inputField.getText();
resultLabel.setText("Entered Text: " + enteredText);
});
// Create a layout for the input field, button, and result label.
VBox root = new VBox(10);
root.getChildren().addAll(inputField, displayButton, resultLabel);
// Create the scene and set it in the stage.
Scene scene = new Scene(root, 300, 200);
primaryStage.setScene(scene);
// Set the title of the window.
primaryStage.setTitle("Text Input App");
// Show the window.
primaryStage.show();
}
}
Explanation:
In the exercise above -
- Import the necessary JavaFX libraries.
- Create a class "Main" that extends "Application" and overrides the "start()" method.
- Inside the "start()" method:
- Create a label to display the entered text (initially showing a default message).
- Create a text input field and a button labeled "Display Text."
- Set an action for the "Display Text" button:
- When the button is clicked, it retrieves the text entered in the input field.
- The entered text is displayed in the label below.
- Organize the input field, button, and label in a vertical layout (VBox) with 10 pixels of spacing between them.
- Create a Scene with the layout, setting the window dimensions to 300x200.
- Set the window's title to "Text Input App."
- Show the window.
Sample Output:
Flowchart:
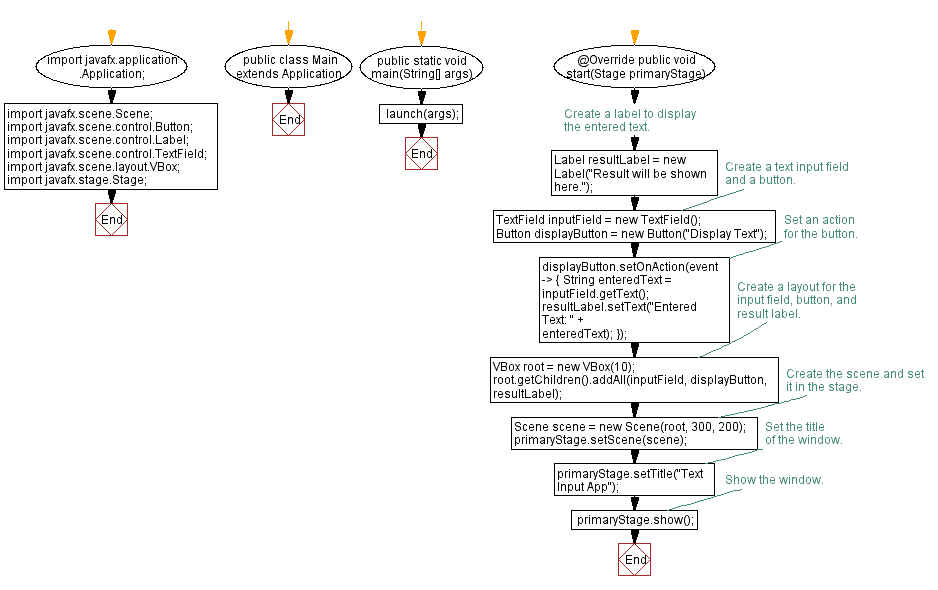
Java Code Editor:
Previous: Basic layout in JavaFX.
Next: ChoiceBox and labels in JavaFX.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics