Image display in JavaFX
JavaFx Basic: Exercise-6 with Solution
Write a JavaFX application that loads an image and displays it in a JavaFX window.
Sample Solution:
JavaFx Code:
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.image.Image;
import javafx.scene.image.ImageView;
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
// Load an image from a file (make sure to provide the correct path to the image).
Image image = new Image("python-tkinter-exercises-practice-solutions.png");
// Create an ImageView to display the image.
ImageView imageView = new ImageView(image);
// Create a layout (StackPane) to display the image.
StackPane root = new StackPane();
root.getChildren().add(imageView);
// Create the scene and set it in the stage.
Scene scene = new Scene(root, 800, 600); // Adjust width and height as needed.
primaryStage.setScene(scene);
// Set the title of the window.
primaryStage.setTitle("Image Display App");
// Show the window.
primaryStage.show();
}
}
Explanation:
In the exercise above -
- Import the necessary JavaFX libraries.
- Create a class "Main" that extends "Application" and overrides the "start()" method.
- Inside the "start()" method:
- Load an image from a file (provide the correct image file path).
- Create an ImageView to display the image.
- Create a layout (StackPane) to display the image.
- Create a Scene with the layout, setting the window dimensions to 800x600 (you can adjust the width and height as needed).
- Set the window's title to "Image Display App."
- Finally, show the window, which will display the loaded image.
Sample Output:
Flowchart:
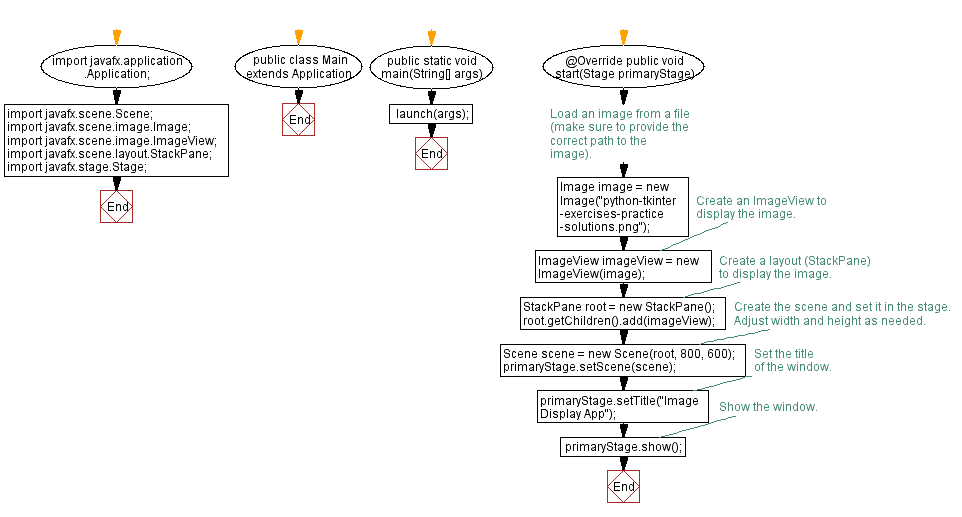
Java Code Editor:
Previous:ChoiceBox and labels in JavaFX.
Next: Simple Calculator in JavaFX.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics