Styling with CSS in JavaFX
JavaFx Basic: Exercise-8 with Solution
Write a JavaFX application that applies CSS styles to change the appearance of buttons and labels.
Sample Solution:
JavaFx Code:
Main.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.Label;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Styled JavaFX App");
VBox root = new VBox(10);
// Create a label and apply a CSS style.
Label styledLabel = new Label("Styled Label");
styledLabel.getStyleClass().add("styled-label");
// Create a button and apply a different CSS style.
Button styledButton = new Button("Styled Button");
styledButton.getStyleClass().add("styled-button");
root.getChildren().addAll(styledLabel, styledButton);
Scene scene = new Scene(root, 300, 200);
scene.getStylesheets().add(getClass().getResource("styles.css").toExternalForm());
primaryStage.setScene(scene);
primaryStage.show();
}
}
styles.css
.styled-label {
-fx-font-size: 20px;
-fx-text-fill: blue;
}
.styled-button {
-fx-font-size: 16px;
-fx-background-color: pink;
-fx-text-fill: red;
-fx-border-color: black;
-fx-border-width: 2px;
-fx-pref-width: 150px;
}
Explanation:
In the exercise above -
- Import the necessary JavaFX libraries.
- Create a class "Main" that extends "Application" and overrides the "start()" method.
- Inside the "start()" method:
- Set the title of the application window to "Styled JavaFX App."
- Create a VBox layout container named root with a spacing of 10 pixels between its children.
- Create a label named 'styledLabel' with the text "Styled Label." It applies a CSS style by adding the "styled-label" style class to it using styledLabel.getStyleClass().add("styled-label").
- Create a button named 'styledButton' with the text "Styled Button." It applies a different CSS style by adding the "styled-button" style class in a similar manner.
- Add both the styled label and button to the root VBox using root.getChildren().addAll(styledLabel, styledButton).
- Create a JavaFX Scene named scene with the root layout, setting its dimensions to 300 pixels in width and 200 pixels in height.
- Add an external CSS stylesheet to the scene using scene.getStylesheets().add(...). The stylesheet is linked via getClass().getResource("styles.css").toExternalForm(), so it should be named "styles.css" and placed in the same directory as your Java code.
- Set the created scene in the primary stage (primaryStage) and show the window.
Sample Output:
Flowchart:
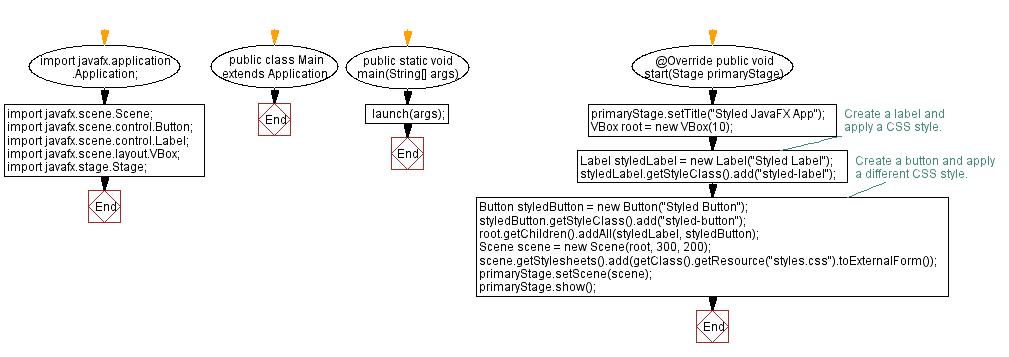
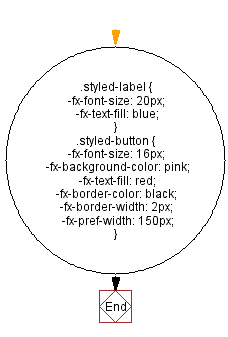
Java Code Editor:
Previous:Simple Calculator in JavaFX.
Next: Event Handling: Creating a Clickable Circle in JavaFX.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics