JavaFX - Stock Trading Simulator
JavaFx Events and Event Handling: Exercise-16 with Solution
Write a JavaFX program to develop a stock trading simulator. Create custom events for stock price changes and use custom event handlers to simulate trading decisions.
Sample Solution:
JavaFx Code:
// StockTradingSimulator.java
import javafx.application.Application;
import javafx.event.Event;
import javafx.event.EventHandler;
import javafx.event.EventType;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class StockTradingSimulator extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Stock Trading Simulator");
Stock stock = new Stock("ABC Company", 100.0);
Trader trader1 = new Trader("Trader 1");
Trader trader2 = new Trader("Trader 2");
stock.setPriceChangeEventHandler(event -> {
trader1.decideTrade(event.getPriceChange());
trader2.decideTrade(event.getPriceChange());
});
Button simulateButton = new Button("Simulate Price Change");
simulateButton.setOnAction(event -> {
double priceChange = Math.random() * 10 - 5; // Simulate a random price change
stock.updatePrice(priceChange);
});
VBox root = new VBox(simulateButton);
Scene scene = new Scene(root, 300, 200);
primaryStage.setScene(scene);
primaryStage.show();
}
public static class Stock {
private String name;
private double price;
private EventHandlerlt;PriceChangeEventgt; priceChangeEventHandler;
public Stock(String name, double initialPrice) {
this.name = name;
this.price = initialPrice;
}
public void setPriceChangeEventHandler(EventHandlerlt;PriceChangeEventgt; handler) {
this.priceChangeEventHandler = handler;
}
public void updatePrice(double priceChange) {
price += priceChange;
if (priceChangeEventHandler != null) {
PriceChangeEvent event = new PriceChangeEvent(priceChange);
priceChangeEventHandler.handle(event);
}
}
}
public static class Trader {
private String name;
public Trader(String name) {
this.name = name;
}
public void decideTrade(double priceChange) {
if (priceChange gt; 0) {
System.out.println(name + " buys stock.");
} else if (priceChange lt; 0) {
System.out.println(name + " sells stock.");
} else {
System.out.println(name + " does not trade.");
}
}
}
public static class PriceChangeEvent extends Event {
public static final EventTypelt;PriceChangeEventgt; PRICE_CHANGE_EVENT = new EventTypelt;gt;("PRICE_CHANGE");
private double priceChange;
public PriceChangeEvent(double priceChange) {
super(PRICE_CHANGE_EVENT);
this.priceChange = priceChange;
}
public double getPriceChange() {
return priceChange;
}
}
}
Explanation:
In the exercise above, there is a simplified stock trading simulator where a stock's price can be changed using a "Simulate Price Change" button. Traders (Trader 1 and Trader 2) make trading decisions based on price changes, and custom events (PriceChangeEvent) are used to notify traders of price changes. The "Trader" class decides whether to buy, sell, or do nothing based on a price change.
Sample Output:
Trader 1 buys stock. Trader 2 buys stock. Trader 1 sells stock. Trader 2 sells stock. Trader 1 buys stock. Trader 2 buys stock. Trader 1 sells stock. Trader 2 sells stock. Trader 1 buys stock. Trader 2 buys stock. Trader 2 sells stock. Trader 1 buys stock. Trader 2 buys stock. Trader 1 buys stock. Trader 2 buys stock ---------------------------- ----------------------------
Flowchart:
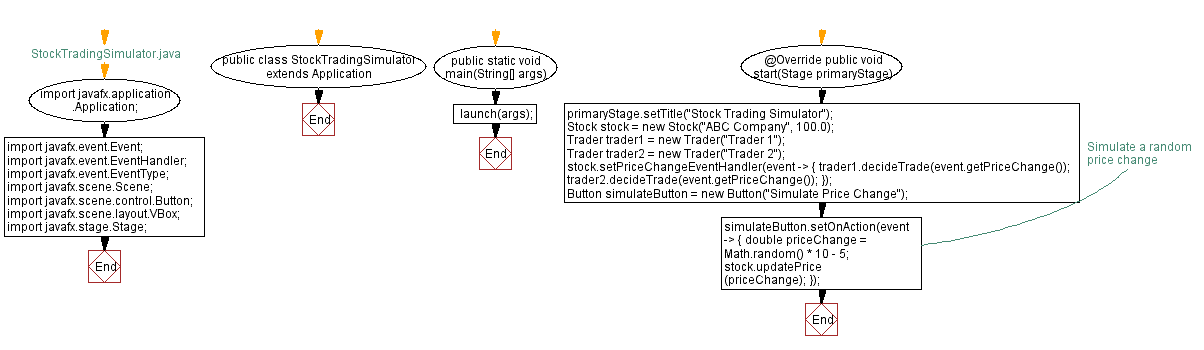

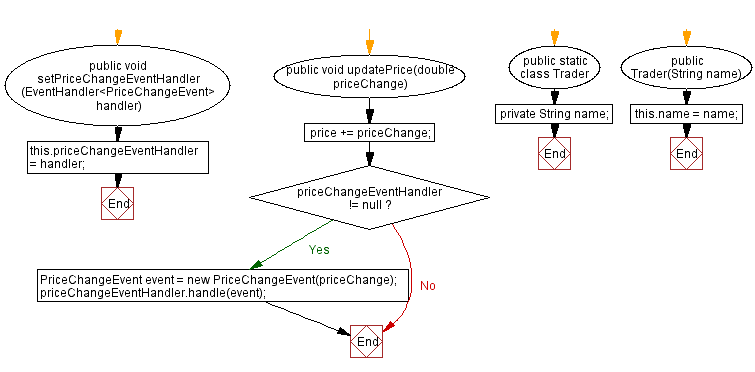
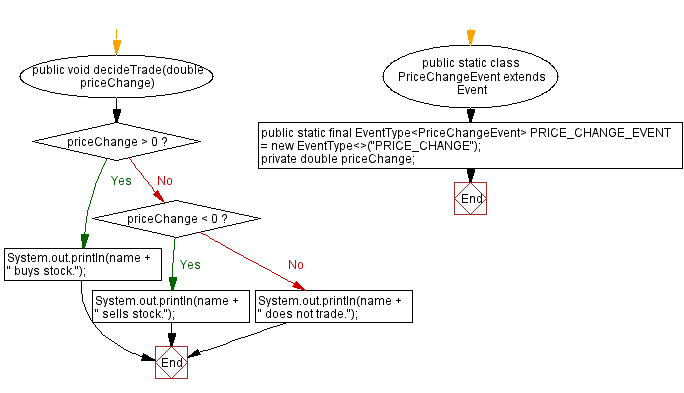
Java Code Editor:
Previous: Simple JavaFX Chat Application.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics