JavaFX Key combination color change
JavaFx Events and Event Handling: Exercise-4 with Solution
Write a JavaFX application that changes the background color of a window based on a combination of keys pressed (e.g., Ctrl+Alt+O changes the background to orange).
Sample Solution:
JavaFx Code:
//Main.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Label; // Import Label
import javafx.scene.layout.StackPane;
import javafx.stage.Stage;
import javafx.scene.input.KeyCode;
import javafx.scene.input.KeyEvent;
public class Main extends Application {
private StackPane root;
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Key Combination Color Change");
root = new StackPane();
Scene scene = new Scene(root, 400, 300);
// Initial background color
root.setStyle("-fx-background-color: white;");
// Label to display the message
Label messageLabel = new Label("Press Ctrl+Alt+O to change the background to orange:");
root.getChildren().add(messageLabel);
// Listen for key presses
scene.addEventHandler(KeyEvent.KEY_PRESSED, event -> {
if (event.isControlDown() && event.isAltDown() && event.getCode() == KeyCode.O) {
// Ctrl+Alt+O changes background to orange
root.setStyle("-fx-background-color: orange;");
}
});
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
In the exercise above, we set up a JavaFX application that listens for key presses. When Ctrl+Alt+O is pressed, the background color changes to orange.
Note: You can add more key combinations and background colors as needed.
Sample Output:
Flowchart:
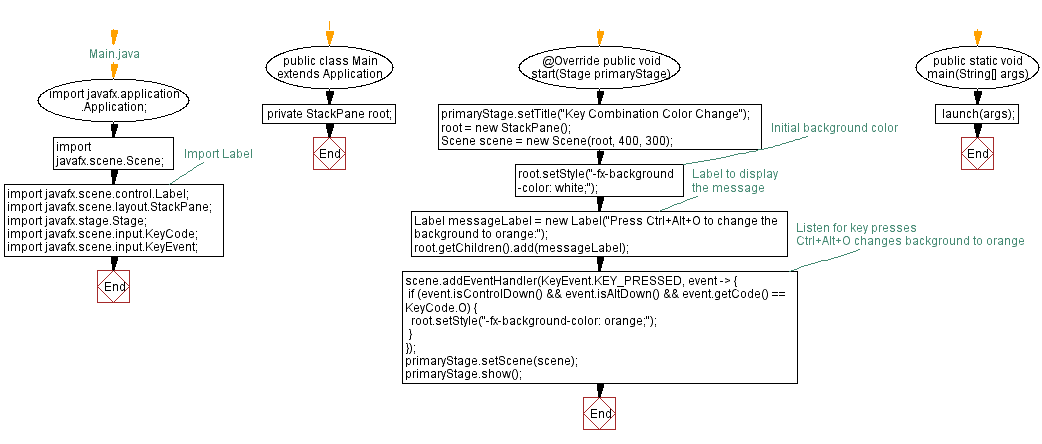
Java Code Editor:
Previous: JavaFX keyboard input example.
Next: JavaFX Button click event.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics