JavaFX styling precedence: CSS vs inline styling override
6. CSS Precedence: External vs Inline Styling
Write a JavaFX program that applies CSS to modify a component's style and then override properties using inline styling. Observe the precedence of styling.
Sample Solution:
JavaFx Code:
// Main.java
import javafx.application.Application;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Main extends Application {
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("CSS Override Example");
// Create a button
Button button = new Button("Styled Button");
// Apply CSS style from an external file
button.getStyleClass().add("styled-button");
// Apply inline styling to override the background color
button.setStyle("-fx-background-color: blue;");
// Add the button to a VBox layout
VBox vbox = new VBox(button);
// Create a scene with the VBox layout
Scene scene = new Scene(vbox, 300, 200);
// Load external CSS file
scene.getStylesheets().add(getClass().getResource("styles.css").toExternalForm());
// Set the scene on the stage and display it
primaryStage.setScene(scene);
primaryStage.show();
}
public static void main(String[] args) {
launch(args);
}
}
/* styles.css */
/* Styling for the button with class 'styled-button' */
.styled-button {
-fx-font-size: 14px;
-fx-padding: 8px 12px;
-fx-background-color: #1E90FF;
-fx-text-fill: white;
-fx-border-color: #1E90FF;
-fx-border-radius: 5px;
}
Explanation:
When we run the above exercise, the button will initially have the styles defined by the .styled-button class from the CSS file. However, inline styling (-fx-background-color: blue;) will override the background color property, resulting in a blue background for the button. This program demonstrates the precedence of inline styling over external CSS styling in JavaFX. You can adjust the styles and observe the changes based on this precedence.
Sample Output:
Flowchart:
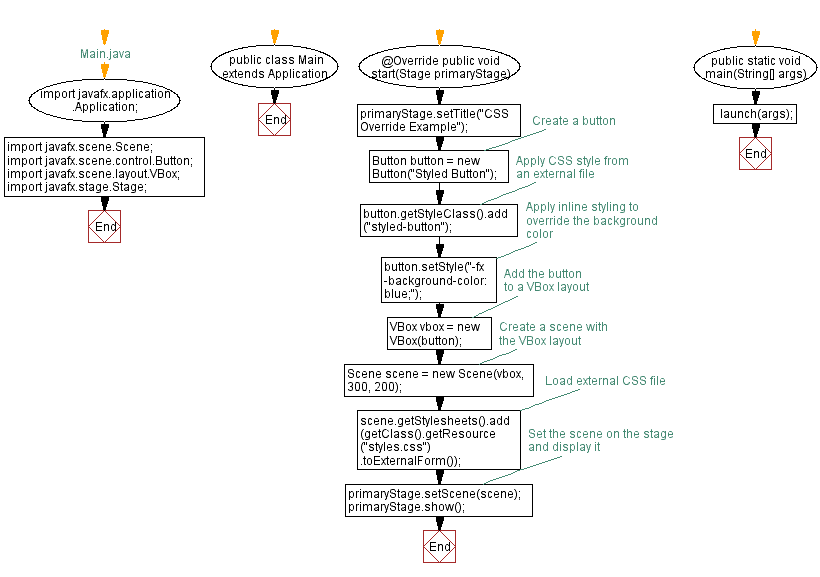
Go to:
PREV : JavaFX Styling with External CSS File.
NEXT : CSS Pseudo-classes for Hover and Focus.
Java Code Editor:
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.