JavaFX TextArea to ListView example
JavaFx User Interface Components: Exercise-19 with Solution
Write a JavaFX application with a TextArea and a button. When text is entered in the TextArea and the button is pressed, display the entered text in a ListView.
Sample Solution:
JavaFx Code:
//Main.java
import javafx.application.Application;
import javafx.collections.FXCollections;
import javafx.scene.Scene;
import javafx.scene.control.Button;
import javafx.scene.control.ListView;
import javafx.scene.control.TextArea;
import javafx.scene.layout.HBox;
import javafx.scene.layout.VBox;
import javafx.stage.Stage;
public class Main extends Application {
public static void main(String[] args) {
launch(args);
}
@Override
public void start(Stage primaryStage) {
primaryStage.setTitle("Text to ListView");
// Create a TextArea for entering text.
TextArea textArea = new TextArea();
textArea.setPrefColumnCount(30);
textArea.setPrefRowCount(5);
// Create a button for adding text to the ListView.
Button addButton = new Button("Add to List");
// Create a ListView to display entered text.
ListView listView = new ListView<>();
addButton.setOnAction(event -> {
String enteredText = textArea.getText();
if (!enteredText.isEmpty()) {
listView.getItems().add(enteredText);
textArea.clear();
}
});
// Create a layout to arrange the components.
HBox inputBox = new HBox(10);
inputBox.getChildren().addAll(textArea, addButton);
VBox root = new VBox(10);
root.getChildren().addAll(inputBox, listView);
// Create the scene and set it in the stage.
Scene scene = new Scene(root, 400, 300);
primaryStage.setScene(scene);
// Set the title of the window.
primaryStage.show();
}
}
In the above JavaFX application, you can input 'text' in the TextArea and click the "Add to List" button. The entered text will be displayed in the ListView below. The ListView dynamically updates with the entered text items.
Sample Output:
Flowchart:
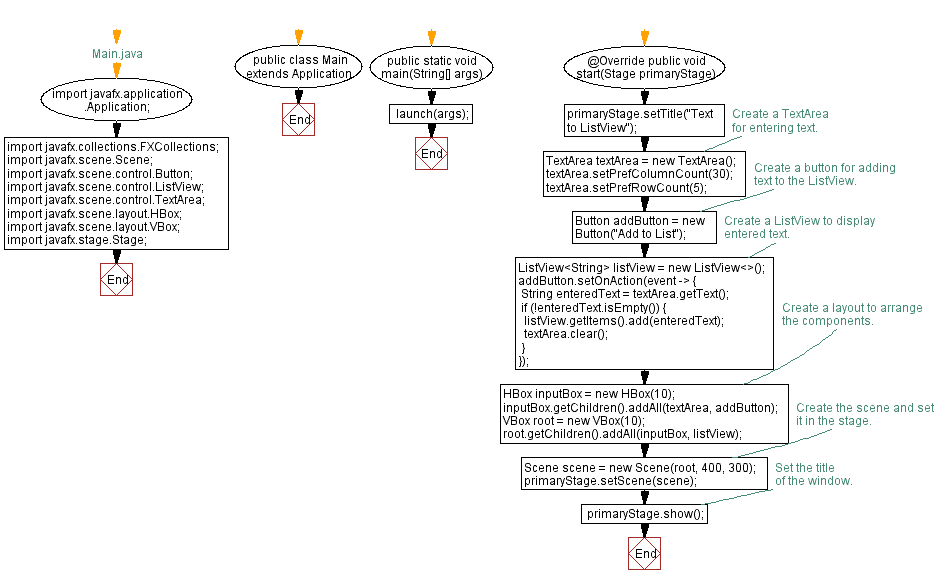
Java Code Editor:
Previous: JavaFX Download manager with ProgressBar.
Next: JavaFX to-do list application with ListView.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics