Concurrent Thread Increment: Java Program to Create and Start Multiple Threads
Java Multithreading: Exercise-1 with Solution
Write a Java program to create and start multiple threads that increment a shared counter variable concurrently.
Sample Solution:
Java Code:
//IncrementThread.java
public class IncrementThread extends Thread {
private Counter counter;
private int incrementsPerThread;
public IncrementThread(Counter counter, int incrementsPerThread) {
this.counter = counter;
this.incrementsPerThread = incrementsPerThread;
}
@Override
public void run() {
for (int i = 0; i < incrementsPerThread; i++) {
counter.increment();
}
}
}
//Counter.java
public class Counter {
private int count = 0;
public synchronized void increment() {
count++;
}
public int getCount() {
return count;
}
}
//Main.java
public class Main {
public static void main(String[] args) {
Counter counter = new Counter();
int numThreads = 6;
int incrementsPerThread = 10000;
IncrementThread[] threads = new IncrementThread[numThreads];
// Create and start the threads
for (int i = 0; i < numThreads; i++) {
threads[i] = new IncrementThread(counter, incrementsPerThread);
threads[i].start();
}
// Wait for all threads to finish
try {
for (IncrementThread thread: threads) {
thread.join();
}
} catch (InterruptedException e) {
e.printStackTrace();
}
// Print the final count
System.out.println("Final count: " + counter.getCount());
}
}
Sample Output:
Final count: 60000
Explanation:
In the above exercise -
- First, we define a "Counter" class that represents a shared counter variable. It has a synchronized increment() method that increments the counter variable by one.
- Next we define an "IncrementThread" class that extends Thread. Each IncrementThread instance increments the shared counter by a specified number of increments.
- In the Main class, we create a 'Counter' object, specify the number of threads and increments per thread, and create an array of 'IncrementThread' objects. We then iterate over the array, creating and starting each thread.
- After starting all the threads, we use the join() method to wait for each thread to finish before proceeding. After all threads have finished, we print the shared counter's final count.
Flowchart:
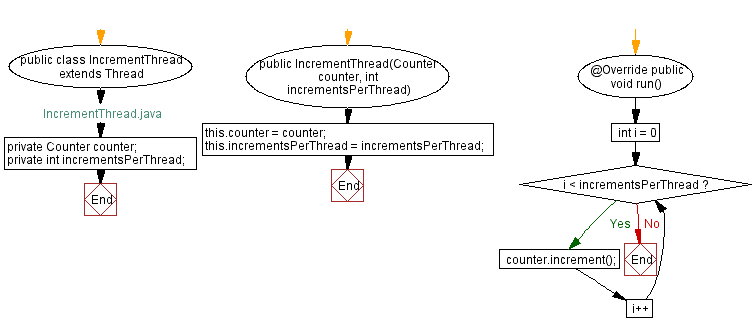
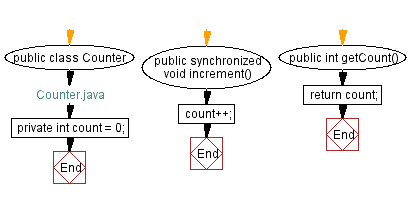
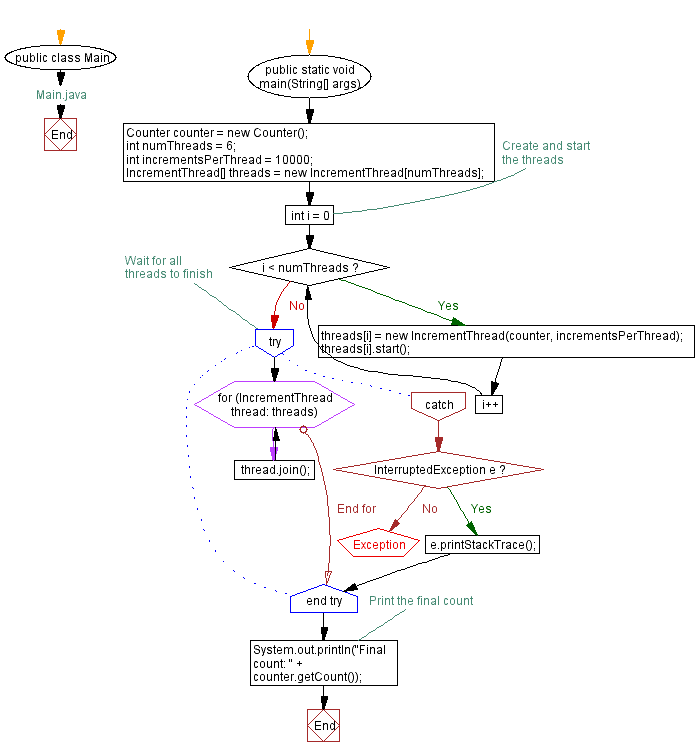
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Java Multithreading Exercises Home.
Next: Java Program with wait() and notify() for Thread Synchronization.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics