Java threading with Phaser: Synchronize multiple threads
Java Multithreading: Exercise-10 with Solution
class Phaser: A reusable synchronization barrier, similar in functionality to CyclicBarrier and CountDownLatch but supporting more flexible usage.
Write a Java program to showcase the usage of the Phaser class for coordination and synchronization of multiple threads.
Sample Solution:
Java Code:
import java.util.concurrent.Phaser;
public class PhaserExercise {
public static void main(String[] args) {
// Create a Phaser with 4 registered parties
Phaser phaser = new Phaser(4);
// Create and start three worker threads
Thread thread1 = new Thread(new Worker(phaser, "Thread 1"));
Thread thread2 = new Thread(new Worker(phaser, "Thread 2"));
Thread thread3 = new Thread(new Worker(phaser, "Thread 3"));
Thread thread4 = new Thread(new Worker(phaser, "Thread 4"));
thread1.start();
thread2.start();
thread3.start();
thread4.start();
// Wait for all threads to complete
phaser.awaitAdvance(phaser.getPhase());
System.out.println("All threads have completed their tasks.");
}
static class Worker implements Runnable {
private final Phaser phaser;
private final String name;
public Worker(Phaser phaser, String name) {
this.phaser = phaser;
this.name = name;
}
@Override
public void run() {
System.out.println(name + " starting phase 1");
phaser.arriveAndAwaitAdvance(); // Wait for all threads to reach this point
// Perform phase 1 tasks
System.out.println(name + " performing phase 1 tasks");
// Wait for all threads to complete phase 1
phaser.arriveAndAwaitAdvance();
System.out.println(name + " starting phase 2");
phaser.arriveAndAwaitAdvance(); // Wait for all threads to reach this point
// Perform phase 2 tasks
System.out.println(name + " performing phase 2 tasks");
// Wait for all threads to complete phase 2
phaser.arriveAndAwaitAdvance();
System.out.println(name + " completed all phases");
phaser.arriveAndDeregister(); // Deregister from the Phaser
}
}
}
Sample Output:
Thread 4 starting phase 1 Thread 1 starting phase 1 Thread 2 starting phase 1 Thread 3 starting phase 1 All threads have completed their tasks. Thread 3 performing phase 1 tasks Thread 4 performing phase 1 tasks Thread 2 performing phase 1 tasks Thread 1 performing phase 1 tasks Thread 1 starting phase 2 Thread 2 starting phase 2 Thread 3 starting phase 2 Thread 4 starting phase 2 Thread 4 performing phase 2 tasks Thread 1 performing phase 2 tasks Thread 3 performing phase 2 tasks Thread 2 performing phase 2 tasks Thread 2 completed all phases Thread 4 completed all phases Thread 3 completed all phases Thread 1 completed all phases
Explanation:
In the above exercise, we create a Phaser with 4 registered parties (threads) and start three worker threads. Each worker thread passes through two phases, and the Phaser ensures that all threads reach specific synchronization points before proceeding to the next phase. Once all threads have completed their tasks, the program outputs a message indicating completion.
The Phaser class provides coordination and synchronization by using methods like arriveAndAwaitAdvance() to wait for all parties to arrive at a specific phase. It also uses arriveAndDeregister() to indicate completion and deregister from the Phaser.
Flowchart:
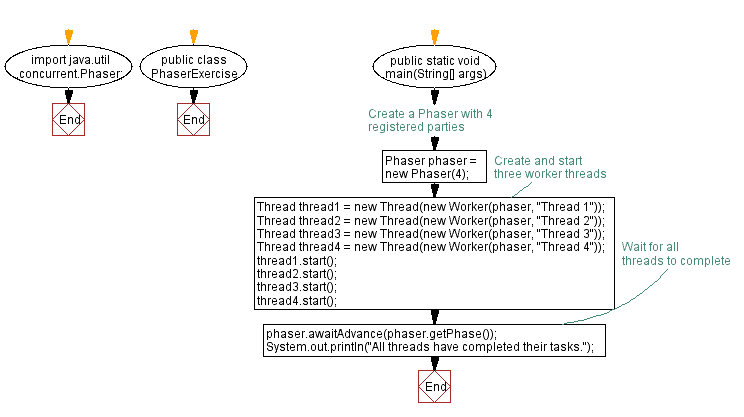
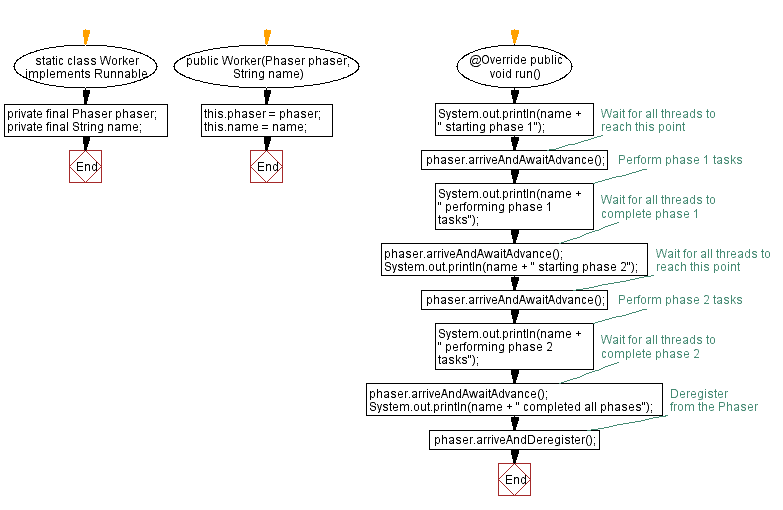
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Implementing Thread-Safe Queue with ConcurrentLinkedQueue.
Next: Communication with Exchanger.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics