Concurrent Read-Write Optimization in Java with StampedLock
Java Multithreading: Exercise-15 with Solution
Write a Java program that utilizes the StampedLock class for optimizing concurrent read-write access to a shared resource.
Sample Solution:
Java Code:
import java.util.concurrent.locks.StampedLock;
public class StampedLockExercise {
public static void main(String[] args) {
SharedResource sharedResource = new SharedResource();
// Start multiple reader threads
for (int i = 0; i < 5; i++) {
new Thread(() -> {
int value = sharedResource.getValue();
System.out.println("Reader thread: " + Thread.currentThread().getName() + ", value: " + value);
}).start();
}
// Start a writer thread
new Thread(() -> {
sharedResource.setValue(42);
System.out.println("Writer thread: " + Thread.currentThread().getName() + " set value to 42");
}).start();
}
static class SharedResource {
private int value;
private final StampedLock lock = new StampedLock();
public int getValue() {
long stamp = lock.tryOptimisticRead(); // Optimistically acquire a read lock
int currentValue = value;
if (!lock.validate(stamp)) { // Check for concurrent write
stamp = lock.readLock(); // Upgrade to a read lock
try {
currentValue = value;
} finally {
lock.unlockRead(stamp); // Release the read lock
}
}
return currentValue;
}
public void setValue(int value) {
long stamp = lock.writeLock(); // Acquire a write lock
try {
this.value = value;
} finally {
lock.unlockWrite(stamp); // Release the write lock
}
}
}
}
Sample Output:
Writer thread: Thread-5 set value to 42 Reader thread: Thread-4, value: 0 Reader thread: Thread-0, value: 0 Reader thread: Thread-1, value: 0 Reader thread: Thread-2, value: 0 Reader thread: Thread-3, value: 0
Explanation:
In the above exercise -
- The "SharedResource" class uses a StampedLock to provide concurrent read-write access to the shared resource while optimizing for reads. Multiple reader threads can concurrently access the value without blocking each other.
- The "getValue()" method demonstrates optimistic read locking. It first acquires an optimistic read lock using tryOptimisticRead(). If the lock is valid, it retrieves the current value without explicitly acquiring a read lock. If the lock is not valid (indicating a concurrent write), it upgrades to a read lock using readLock() and retrieves the value. Finally, it releases the read lock using unlockRead().
- The "setValue()" method acquires a write lock using writeLock() to update the value. Once the update is complete, it releases the write lock using unlockWrite().
- In the main() method, multiple reader threads are started concurrently, each accessing the shared resource using getValue(). Additionally, a writer thread sets the value using setValue().
- When you run this program, you'll see that the reader threads can access the value concurrently without blocking each other. In addition, the writer thread can update the value exclusively.
Flowchart:
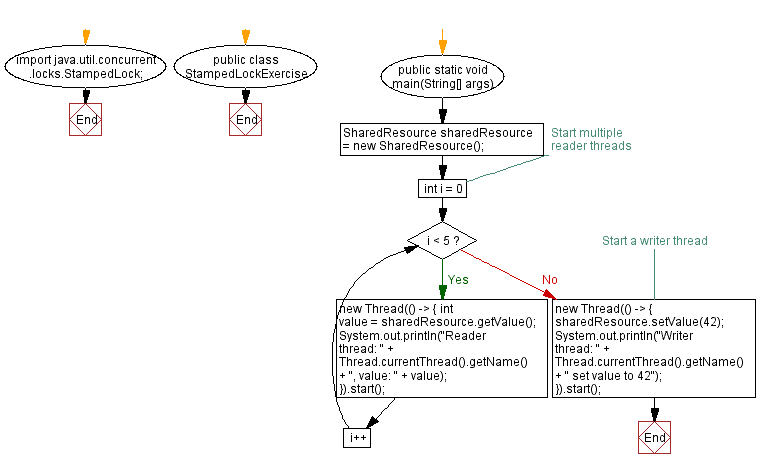
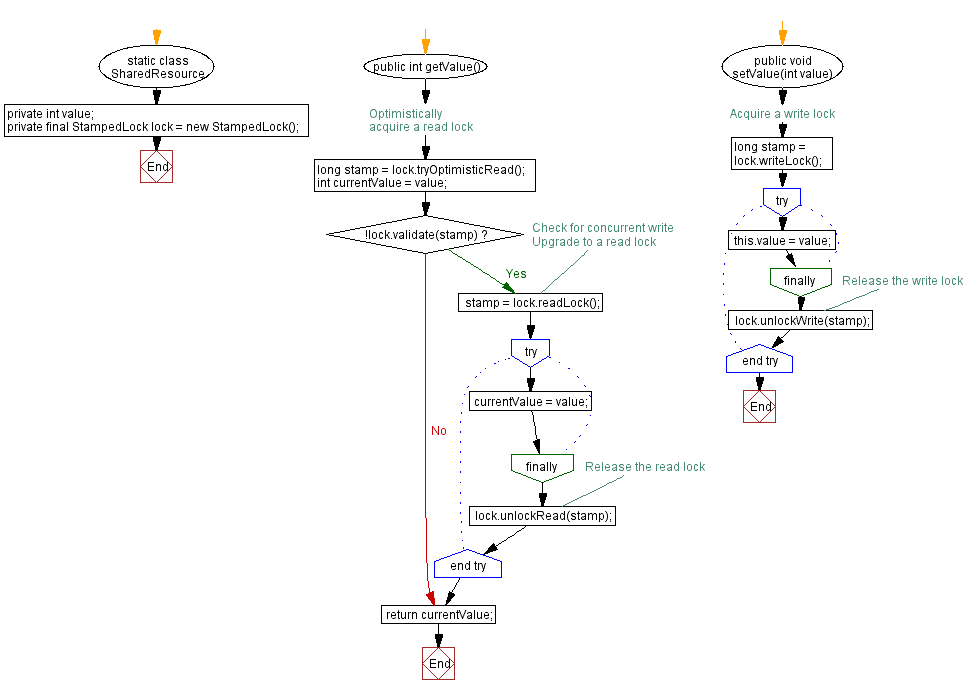
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Parallel Recursive Task Execution in Java with ForkJoinPool.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics