Java Program: Synchronizing Threads with ReentrantLock for Shared Resource
Java Multithreading: Exercise-3 with Solution
A reentrant mutual exclusion Lock with the same basic behavior and semantics as the implicit monitor lock accessed using synchronized methods and statements, but with extended capabilities.
A ReentrantLock is owned by the thread last successfully locking, but not yet unlocking it. A thread invoking lock will return, successfully acquiring the lock, when the lock is not owned by another thread. The method will return immediately if the current thread already owns the lock. This can be checked using methods isHeldByCurrentThread(), and getHoldCount().
Write a Java program that uses the ReentrantLock class to synchronize access to a shared resource among multiple threads.
Sample Solution:
Java Code:
// SharedResourceExercise.java
import java.util.concurrent.locks.Lock;
import java.util.concurrent.locks.ReentrantLock;
public class SharedResourceExercise {
private static final int NUM_THREADS = 3;
private static final int NUM_ITERATIONS = 5;
public static void main(String[] args) {
Lock lock = new ReentrantLock();
SharedResource sharedResource = new SharedResource();
Thread[] threads = new Thread[NUM_THREADS];
for (int i = 0; i < NUM_THREADS; i++) {
threads[i] = new Thread(new Worker(lock, sharedResource));
threads[i].start();
}
try {
for (Thread thread: threads) {
thread.join();
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
static class Worker implements Runnable {
private Lock lock;
private SharedResource sharedResource;
public Worker(Lock lock, SharedResource sharedResource) {
this.lock = lock;
this.sharedResource = sharedResource;
}
public void run() {
for (int i = 0; i < NUM_ITERATIONS; i++) {
lock.lock();
try {
sharedResource.doWork();
} finally {
lock.unlock();
}
}
}
}
static class SharedResource {
public void doWork() {
String threadName = Thread.currentThread().getName();
System.out.println("Thread-> " + threadName + " is performing work.");
// Perform work on the shared resource
}
}
}
Sample Output:
Thread-> Thread-0 is performing work. Thread-> Thread-0 is performing work. Thread-> Thread-0 is performing work. Thread-> Thread-0 is performing work. Thread-> Thread-0 is performing work. Thread-> Thread-1 is performing work. Thread-> Thread-1 is performing work. Thread-> Thread-1 is performing work. Thread-> Thread-1 is performing work. Thread-> Thread-1 is performing work. Thread-> Thread-2 is performing work. Thread-> Thread-2 is performing work. Thread-> Thread-2 is performing work. Thread-> Thread-2 is performing work. Thread-> Thread-2 is performing work.
Explanation:
In the above exercise -
- The "SharedResourceExercise" class represents the main program. It creates an instance of the ReentrantLock class as the lock object and an instance of the "SharedResource" class as the shared resource object.
- The "Worker" class implements the Runnable interface and represents the worker thread. Each worker thread acquires the lock using the lock() method before accessing the shared resource's doWork() method. After performing the work, the thread releases the lock using the unlock() method.
- The "SharedResource" class represents shared resources. In this example, the doWork() method simulates work on the shared resource.
- In the main() method, we create an array of worker threads, start them concurrently, and wait for them to finish using the join() method.
Flowchart:
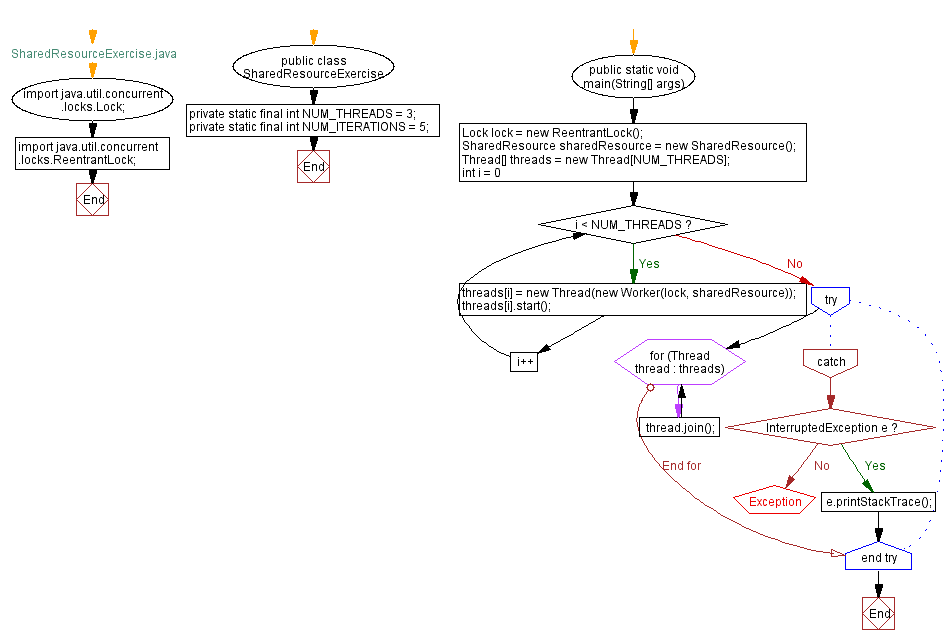
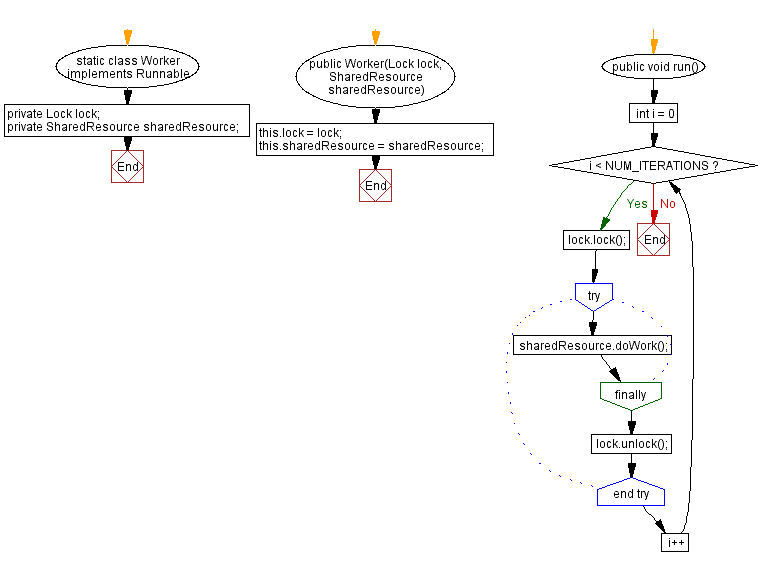
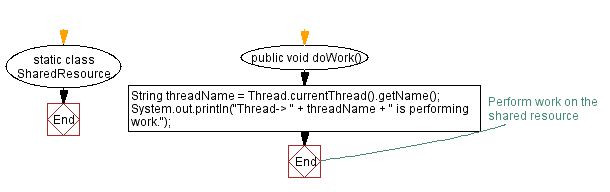
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Java Program with wait() and notify() for Thread Synchronization.
Next: Thread Synchronization with Semaphores.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics