Java Program: Thread Synchronization with CyclicBarrier
Java Multithreading: Exercise-5 with Solution
A synchronization aid that allows a set of threads to all wait for each other to reach a common barrier point. CyclicBarriers are useful in programs involving a fixed sized party of threads that must occasionally wait for each other. The barrier is called cyclic because it can be re-used after the waiting threads are released.
A CyclicBarrier supports an optional Runnable command that is run once per barrier point, after the last thread in the party arrives, but before any threads are released. This barrier action is useful for updating shared-state before any of the parties continue.
Write a Java program to showcase the usage of the CyclicBarrier class for thread synchronization.
Sample Solution:
Java Code:
import java.util.concurrent.BrokenBarrierException;
import java.util.concurrent.CyclicBarrier;
public class CyclicBarrierExercise {
private static final int NUM_THREADS = 3;
private static final CyclicBarrier barrier = new CyclicBarrier(NUM_THREADS, new BarrierAction());
public static void main(String[] args) {
Thread[] threads = new Thread[NUM_THREADS];
for (int i = 0; i < NUM_THREADS; i++) {
threads[i] = new Thread(new Worker());
threads[i].start();
}
try {
for (Thread thread: threads) {
thread.join();
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
static class Worker implements Runnable {
public void run() {
try {
System.out.println("Thread " + Thread.currentThread().getName() + " is waiting at the barrier.");
barrier.await();
// Perform work after reaching the barrier
System.out.println("Thread " + Thread.currentThread().getName() + " has crossed the barrier and continued execution.");
} catch (InterruptedException | BrokenBarrierException e) {
e.printStackTrace();
}
}
}
static class BarrierAction implements Runnable {
public void run() {
System.out.println("Barrier reached! All threads have arrived at the barrier.");
}
}
}
Sample Output:
Thread Thread-2 is waiting at the barrier. Thread Thread-0 is waiting at the barrier. Thread Thread-1 is waiting at the barrier. Barrier reached! All threads have arrived at the barrier. Thread Thread-0 has crossed the barrier and continued execution. Thread Thread-1 has crossed the barrier and continued execution. Thread Thread-2 has crossed the barrier and continued execution.
Explanation:
In the above exercise -
- The "CyclicBarrierExample" class represents the main program. It creates an instance of the CyclicBarrier class with the number of parties set to NUM_THREADS, and a BarrierAction object as the barrier action to be executed when all threads reach the barrier.
- The "Worker" class implements the Runnable interface and represents the worker thread. Each worker thread waits at the barrier using await(). Once all threads reach the barrier, the barrier action is executed, and all threads continue with their execution.
- The BarrierAction class represents the barrier action to be executed when all threads reach the barrier. In this example, it simply prints a message indicating that the barrier has been reached.
- In the main() method, we create an array of worker threads, start them concurrently, and wait for them to finish using the join() method.
Flowchart:
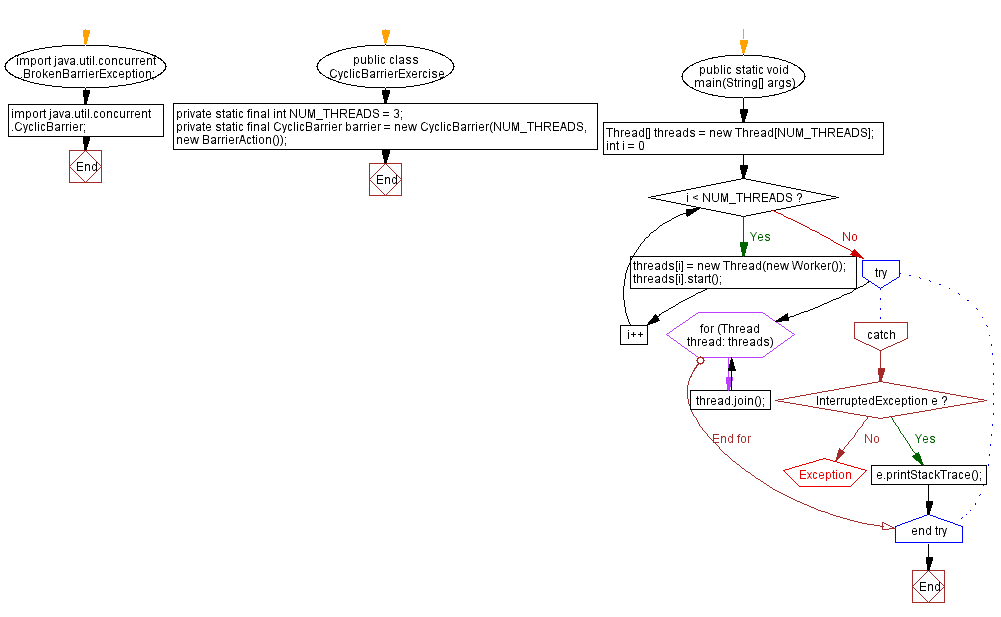
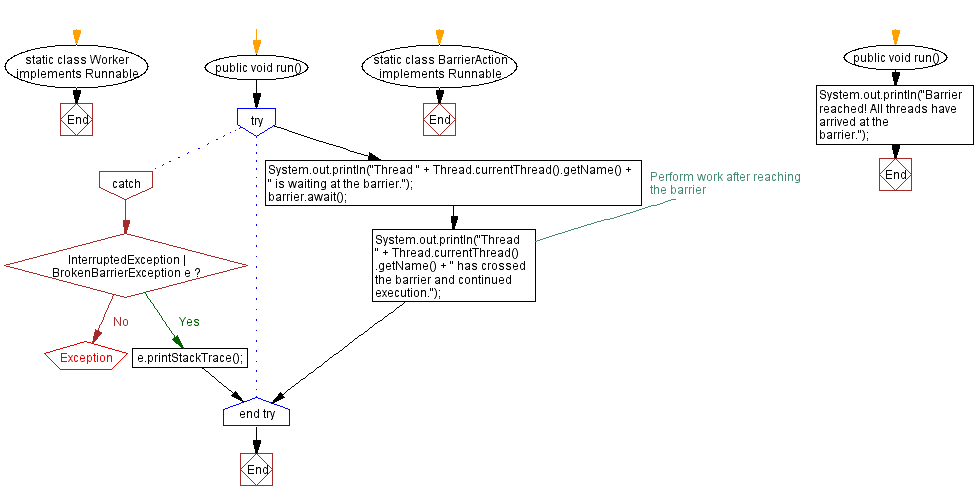
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Thread Synchronization with Semaphores.
Next: Thread Synchronization with CountDownLatch.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics