Java Program: Thread Synchronization with CountDownLatch
Java Multithreading: Exercise-6 with Solution
A synchronization aid that allows one or more threads to wait until a set of operations being performed in other threads completes.
A CountDownLatch is initialized with a given count. The await methods block until the current count reaches zero due to invocations of the countDown() method, after which all waiting threads are released and any subsequent invocations of await return immediately. This is a one-shot phenomenon -- the count cannot be reset. If you need a version that resets the count, consider using a CyclicBarrier.
A CountDownLatch is a versatile synchronization tool and can be used for a number of purposes. A CountDownLatch initialized with a count of one serves as a simple on/off latch, or gate: all threads invoking await wait at the gate until it is opened by a thread invoking countDown(). A CountDownLatch initialized to N can be used to make one thread wait until N threads have completed some action, or some action has been completed N times.
A useful property of a CountDownLatch is that it doesn't require that threads calling countDown wait for the count to reach zero before proceeding, it simply prevents any thread from proceeding past an await until all threads could pass.
Write a Java program that uses the CountDownLatch class to synchronize the start and finish of multiple threads.
Sample Solution:
Java Code:
import java.util.concurrent.CountDownLatch;
public class CountDownLatchExercise {
private static final int NUM_THREADS = 3;
private static final CountDownLatch startLatch = new CountDownLatch(1);
private static final CountDownLatch finishLatch = new CountDownLatch(NUM_THREADS);
public static void main(String[] args) {
Thread[] threads = new Thread[NUM_THREADS];
for (int i = 0; i < NUM_THREADS; i++) {
threads[i] = new Thread(new Worker());
threads[i].start();
}
// Allow threads to start simultaneously
startLatch.countDown();
try {
finishLatch.await();
} catch (InterruptedException e) {
e.printStackTrace();
}
System.out.println("All threads have finished their work.");
}
static class Worker implements Runnable {
public void run() {
try {
startLatch.await();
// Perform work
System.out.println("Thread " + Thread.currentThread().getName() + " has finished its work.");
} catch (InterruptedException e) {
e.printStackTrace();
} finally {
finishLatch.countDown();
}
}
}
}
Sample Output:
Thread Thread-0 has finished its work. Thread Thread-1 has finished its work. Thread Thread-2 has finished its work. All threads have finished their work.
Explanation:
In the above exercise -
- The "CountDownLatchExample" class represents the main program. It creates two instances of CountDownLatch: startLatch with an initial count of 1 to synchronize the start of all threads, and finishLatch with an initial count equal to the number of threads to synchronize the finish of all threads.
- The "Worker" class implements the Runnable interface and represents the worker thread. Each worker thread waits at the startLatch using the await() method until the latch is counted down to zero. Once the latch is counted down, all threads start simultaneously. After completing their work, each thread counts down the finishLatch using the countDown() method.
- In the main() method, we create an array of worker threads, start them concurrently, and allow them to start simultaneously. We do this by counting down the startLatch using the countDown() method. We then wait for all threads to finish their work by using the await() method on the finishLatch. Finally, we print a message indicating that all threads have finished their work.
- The program output shows the completion of each thread's work and the final message indicates that all threads have finished.
Flowchart:
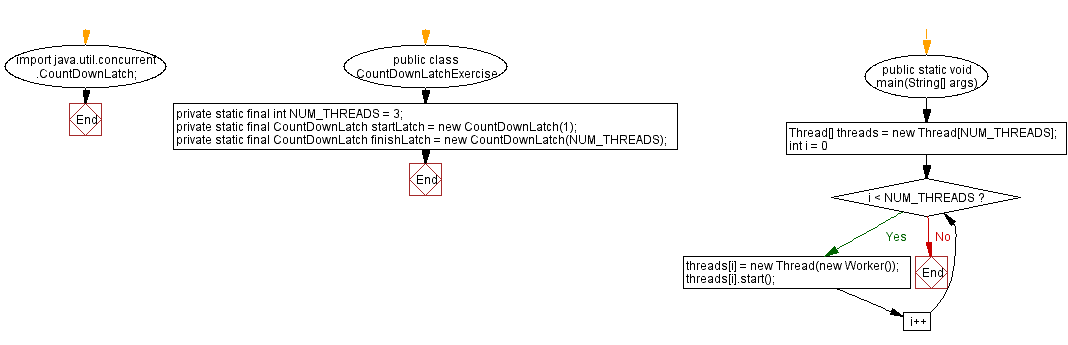
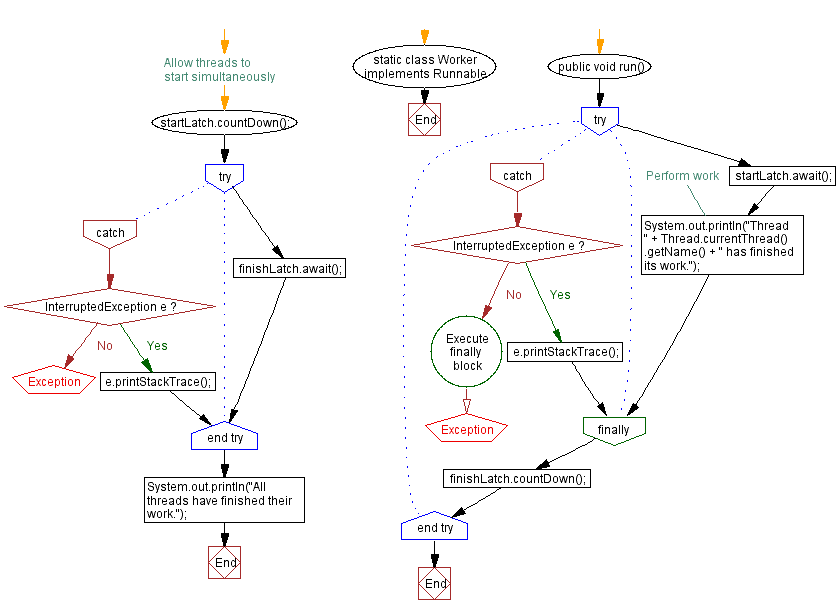
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Thread Synchronization with CyclicBarrier.
Next: Concurrent Read-Write Access with ReadWriteLock.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics