Java Program: Concurrent Map Access with ConcurrentHashMap
Java Multithreading: Exercise-8 with Solution
ConcurrentHashMap:
A hash table supporting full concurrency of retrievals and high expected concurrency for updates. This class obeys the same functional specification as Hashtable, and includes versions of methods corresponding to each method of Hashtable. However, even though all operations are thread-safe, retrieval operations do not entail locking, and there is not any support for locking the entire table in a way that prevents all access. This class is fully interoperable with Hashtable in programs that rely on its thread safety but not on its synchronization details.
Write a Java program demonstrating how to access a map concurrently using the ConcurrentHashMap class.
Sample Solution:
Java Code:
import java.util.concurrent.ConcurrentHashMap;
public class ConcurrentHashMapExercise {
public static void main(String[] args) {
ConcurrentHashMap < String, Integer > map = new ConcurrentHashMap < > ();
// Create and start the writer threads
Thread writerThread1 = new Thread(new Writer(map, "Thread-1", 1));
Thread writerThread2 = new Thread(new Writer(map, "Thread-2", 2));
writerThread1.start();
writerThread2.start();
// Create and start the reader threads
Thread readerThread1 = new Thread(new Reader(map, "Thread-1"));
Thread readerThread2 = new Thread(new Reader(map, "Thread-2"));
readerThread1.start();
readerThread2.start();
}
static class Writer implements Runnable {
private ConcurrentHashMap < String, Integer > map;
private String threadName;
private int value;
public Writer(ConcurrentHashMap < String, Integer > map, String threadName, int value) {
this.map = map;
this.threadName = threadName;
this.value = value;
}
public void run() {
for (int i = 0; i < 5; i++) {
map.put(threadName, value);
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
static class Reader implements Runnable {
private ConcurrentHashMap < String, Integer > map;
private String threadName;
public Reader(ConcurrentHashMap < String, Integer > map, String threadName) {
this.map = map;
this.threadName = threadName;
}
public void run() {
for (int i = 0; i < 5; i++) {
Integer value = map.get(threadName);
System.out.println("Thread " + threadName + " read value: " + value);
try {
Thread.sleep(1000);
} catch (InterruptedException e) {
e.printStackTrace();
}
}
}
}
}
Sample Output:
Thread Thread-1 read value: 1 Thread Thread-2 read value: 2 Thread Thread-1 read value: 1 Thread Thread-2 read value: 2 Thread Thread-2 read value: 2 Thread Thread-1 read value: 1 Thread Thread-1 read value: 1 Thread Thread-2 read value: 2 Thread Thread-1 read value: 1 Thread Thread-2 read value: 2
Explanation:
In the above exercise:
- The "ConcurrentHashMapExercise" class represents the main program. It creates an ConcurrentHashMap instance called map.
- The "Writer" class implements the Runnable interface and represents a writer thread. Each writer thread puts key-value pairs into the map using the put() method. In this example, each writer thread puts its thread name as the key and an incrementing value as the value.
- The "Reader" class implements the Runnable interface and represents a reader thread. Each reader thread gets the value associated with its thread name from the map using the get() method. It then prints the value.
- In the main() method, we create and start two writer threads and two reader threads. Writers put key-value pairs into the map, and readers get the values from the map. Since ConcurrentHashMap supports concurrent access, writers and readers can access the map simultaneously without explicit synchronization.
Flowchart:
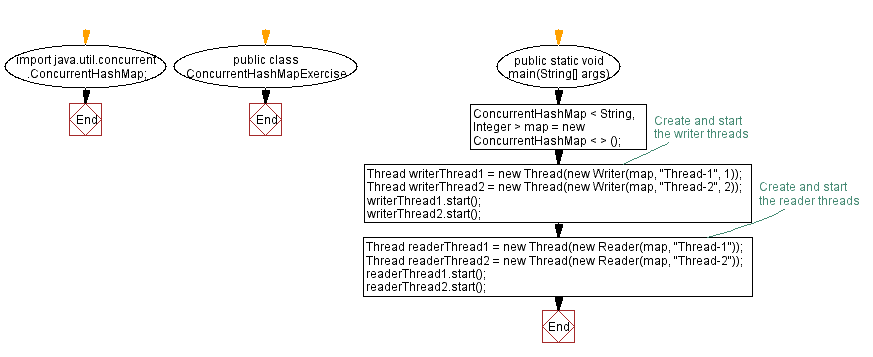
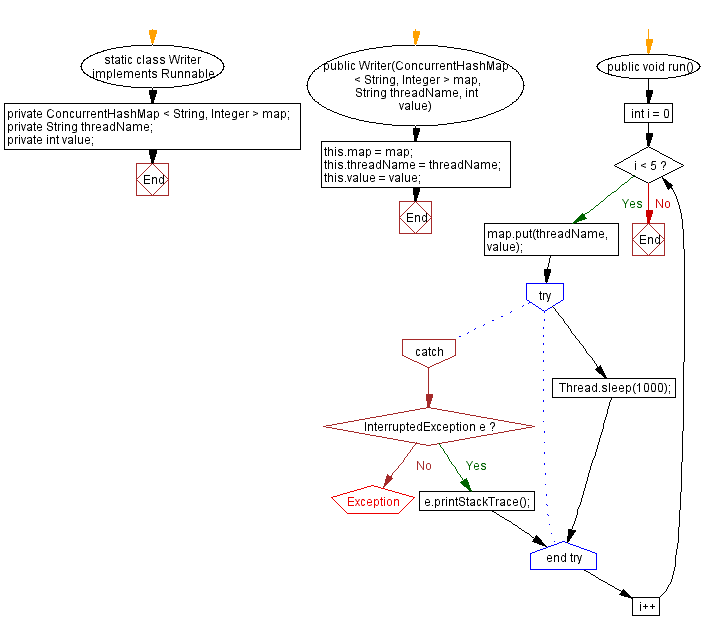
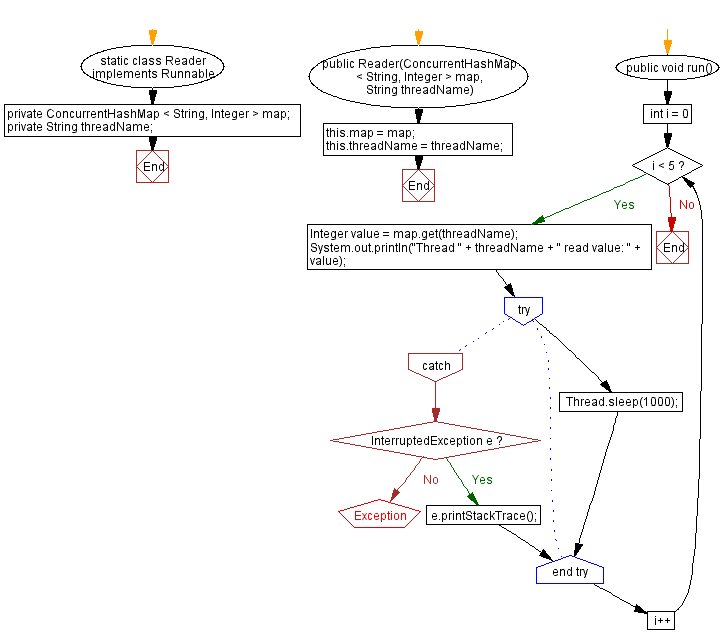
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Concurrent Read-Write Access with ReadWriteLock.
Next: Implementing Thread-Safe Queue with ConcurrentLinkedQueue.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics