Java: Concatenate a given string to the end of another string
Java String: Exercise-7 with Solution
Write a Java program to concatenate a given string to the end of another string.
Visual Presentation:
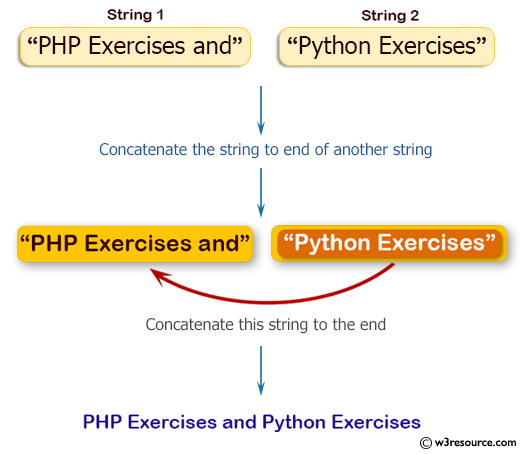
Sample Solution:
Java Code:
// Define a public class named Exercise7.
public class Exercise7 {
// Define the main method.
public static void main(String[] args) {
// Declare and initialize two string variables, str1 and str2.
String str1 = "PHP Exercises and ";
String str2 = "Python Exercises";
// Print the first string.
System.out.println("String 1: " + str1);
// Print the second string.
System.out.println("String 2: " + str2);
// Concatenate the two strings together and store the result in str3.
String str3 = str1.concat(str2);
// Display the newly concatenated string.
System.out.println("The concatenated string: " + str3);
}
}
Sample Output:
String 1: PHP Exercises and String 2: Python Exercises The concatenated string: PHP Exercises and Python Exercises
Flowchart:
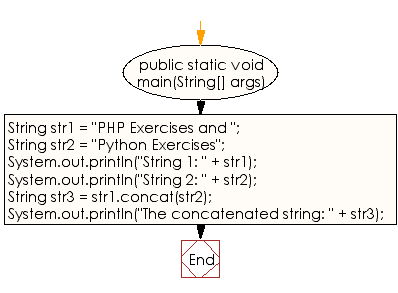
Java Code Editor:
Improve this sample solution and post your code through Disqus
Previous: Write a java program to compare two strings lexicographically, ignoring case differences.
Next: Write a Java program to test if a given string contains the specified sequence of char values.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics