PHP script: Limit maximum concurrent sessions to 3
PHP Cookies and Sessions: Exercise-13 with Solution
Write a PHP script to limit the maximum number of concurrent sessions for a user to 3.
Sample Solution:
PHP Code :
<?php
// Set the session save path
session_save_path('i:/custom/');
session_start();
$maxSessions = 3; // Maximum number of concurrent sessions allowed for a user
if (!isset($_SESSION['session_count'])) {
$_SESSION['session_count'] = 1;
} else {
$_SESSION['session_count']++;
if ($_SESSION['session_count'] > $maxSessions) {
session_unset();
session_destroy();
echo "Maximum session limit exceeded. Please log in again.";
exit;
}
}
echo "Session active. Session count: " . $_SESSION['session_count'];
?>
Sample Output:
Session active. Session count: 1 ----------------------------------------------------- Session active. Session count: 2 ----------------------------------------------------- Session active. Session count: 3 ----------------------------------------------------- Maximum session limit exceeded. Please log in again.
Explanation:
In the above exercise -
- We start the session using session_start() to initialize the session.
- Define the maximum number of concurrent sessions allowed for a user using the variable $maxSessions.
- Check if the session variable 'session_count' is set. If it is not set, initialize it to 1, indicating the first session for the user.
- If the 'session_count' session variable is already set, we increment its value by 1.
- Then check if the updated 'session_count' value exceeds the maximum allowed sessions ($maxSessions). If it does, we unset all session variables using session_unset(), destroy the session using session_destroy(), display a message indicating the maximum session limit has been exceeded, and exit the script.
- If the session count is within the limit, display a message indicating that the session is active along with the current session count.
When a user accesses the script, it checks the current session count for the user. If the count is within the limit, the session continues and the count is incremented. If the count exceeds the limit, the session is destroyed, and a message is displayed indicating the maximum session limit has been exceeded.
Flowchart:
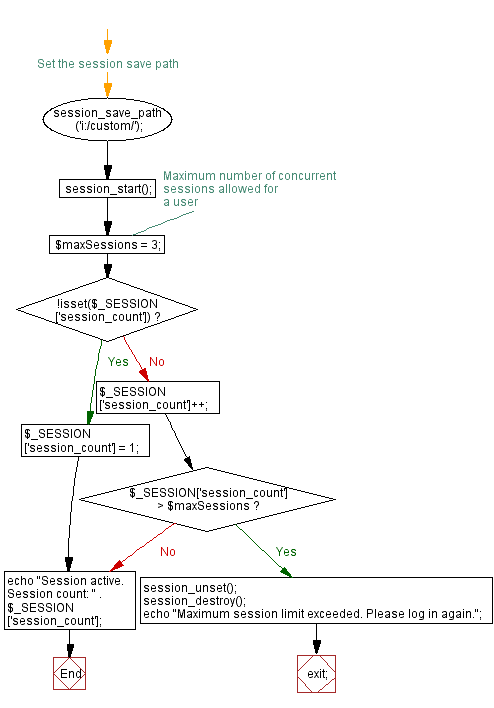
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: PHP script: Limit maximum concurrent sessions to 3.
Next: Regenerate session ID for security.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics