Set a secure PHP cookie for an encrypted connection
PHP Cookies and Sessions: Exercise-7 with Solution
Write a PHP script to set a secure cookie that can only be transmitted over an encrypted connection.
Sample Solution:
PHP Code :
<?php
$cookieName = "my_Cookie";
$cookieValue = "Example_cookie_value";
$expirationTime = time() + 3600; // current time + 1 hour
$secureOnly = true; // Set the cookie to be transmitted only over HTTPS
setcookie($cookieName, $cookieValue, $expirationTime, "/", "", $secureOnly, true);
echo "Secure cookie named 'my_Cookie' has been set.";
?>
Sample Output:
Secure cookie named 'my_Cookie' has been set.
Explanation:
In the above exercise -
- $cookieName: The cookie name, set to "myCookie".
- $cookieValue: The cookie value, set to "example value".
- $expirationTime: The cookie expiration time, calculated as the current time (time()) plus 1 hour (3600 seconds).
- $secureOnly: A boolean variable set to true to indicate that the cookie should only be transmitted over an encrypted connection (HTTPS).
We then use the setcookie() function to set the cookie with the specified name, value, expiration time, and secure flag. In order to make the cookie accessible on the entire domain and its subdomains, the last two parameters are set to / and "".
Flowchart:
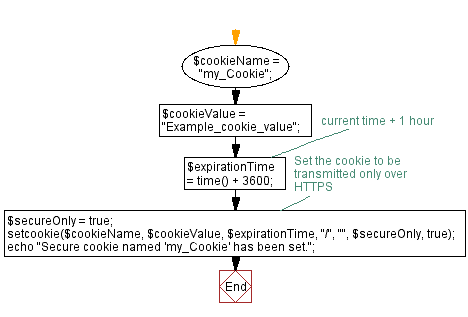
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Destroy PHP session and unset all session variables.
Next: PHP script to check cookie existence and display a message.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics