PHP Exception Handling: Basic usage of try-catch blocks
PHP Exception Handling: Exercise-1 with Solution
Write a PHP program that demonstrates the basic usage of try-catch blocks to handle exceptions.
Sample Solution:
PHP Code :
<?php
try {
// Code that may throw an exception
$numerator = 100;
$denominator = 0;
if ($denominator === 0) {
throw new Exception("Division by zero is not allowed.");
}
$result = $numerator / $denominator;
echo "Result: " . $result;
} catch (Exception $e)
{
// Exception handling code
echo "An error occurred: " . $e->getMessage();
}
?>
Sample Output:
An error occurred: Division by zero is not allowed.
Explanation:
In the above exercise, we have a division operation where the denominator is intentionally set to 0 to cause a division by zero exception. The code is wrapped inside a try block, and if an exception occurs within the try block, it is caught by the catch block. The catch block then handles the exception by displaying an error message using $e-<getMessage().
Flowchart:
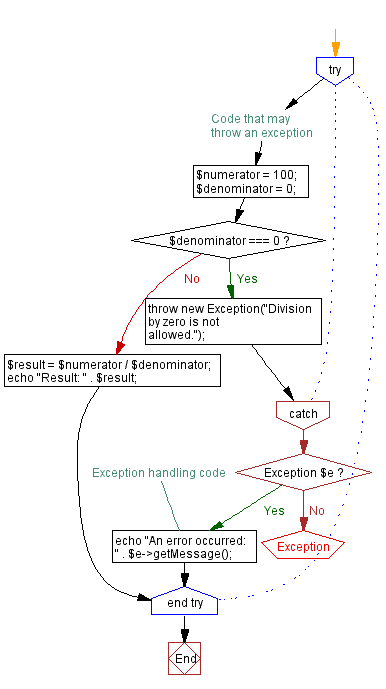
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: PHP Exception Handling Exercises Home
Next: Creating and throwing custom exceptions.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics