PHP exception handling: Handling specific exceptions
PHP Exception Handling: Exercise-10 with Solution
Write a PHP program that uses a try-catch block to handle a specific exception and performs specific actions based on the exception type.
Sample Solution:
PHP Code :
<?php
class CustomException extends Exception {
public function __construct($message, $code = 0, Throwable $previous = null) {
parent::__construct($message, $code, $previous);
}
public function __toString() {
return __CLASS__ . ": [{$this->code}]: {$this->message}\n";
}
}
try {
// Code that may throw a specific exception
$number = "abc";
if (!is_numeric($number)) {
throw new CustomException("Invalid number.");
}
$result = 200 / $number;
echo "Result: " . $result;
} catch (CustomException $e) {
// Handle CustomException
echo "CustomException: " . $e->getMessage();
// Perform specific actions for CustomException
// ...
} catch (Exception $e) {
// Handle generic Exception
echo "Exception: " . $e->getMessage();
}
?>
Sample Output:
CustomException: Invalid number
Explanation:
In the above exercise,
- We have a try block that contains code that throws a specific exception. We check if the $number is numeric using is_numeric() and throw a CustomException if it's not.
- The first catch block catches the CustomException specifically and handles it separately. It displays an appropriate error message using $e->getMessage() and can perform specific actions for the CustomException.
- The second catch block catches a generic Exception if another type of exception occurs. It displays an appropriate error message using $e->getMessage().
Flowchart:
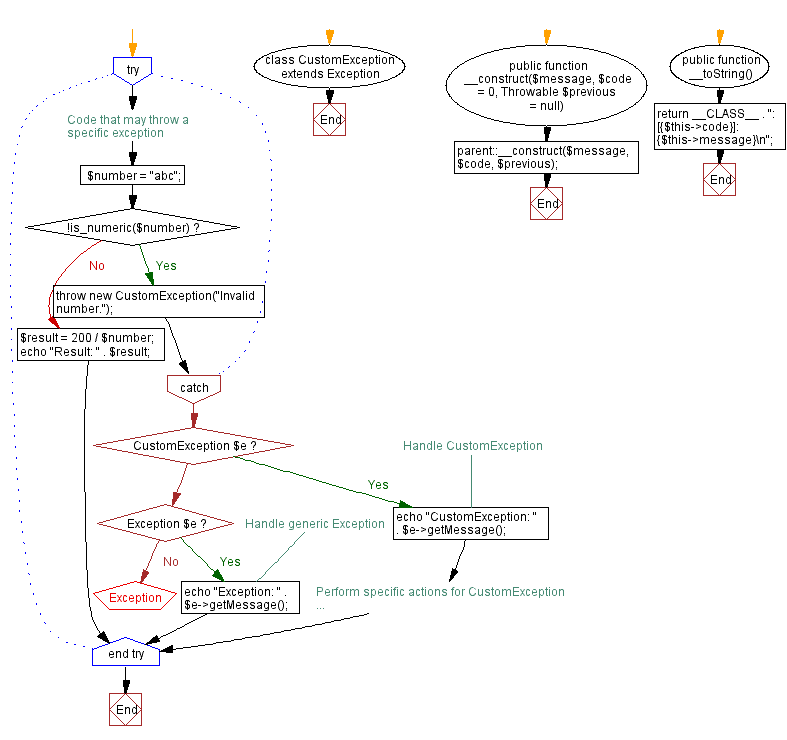
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics