PHP Custom Exception Class: Creating and throwing custom exceptions
PHP Exception Handling: Exercise-2 with Solution
Write a PHP program that creates a custom exception class in PHP and throw an instance of it within a try block.
Sample Solution:
PHP Code :
<?php
class CustomException extends Exception {
public function __construct($message, $code = 0, Throwable $previous = null) {
parent::__construct($message, $code, $previous);
}
public function __toString() {
return __CLASS__ . ": [{$this->code}]: {$this->message}\n";
}
}
try {
// Code that may throw a custom exception
$roll_no = -15;
if ($roll_no < 0) {
throw new CustomException("Roll number cannot be negative.");
}
echo "Roll number: " . $age;
} catch (CustomException $e) {
// Custom exception handling code
echo "An error occurred: " . $e->getMessage();
}
?>
Sample Output:
An error occurred: Roll number cannot be negative.
Explanation:
In the above exercise,
- In this example, we create a custom exception class CustomException that extends the base Exception class. The custom exception class has a constructor that calls the parent constructor and overrides the __toString() method to provide a custom string representation of the exception.
- Inside the try block, we have a check for a negative age. If the condition is true, we throw an instance of the CustomException class with the message "Age cannot be negative."
- The catch block specifically catches instances of the CustomException class, allowing us to handle the custom exception separately. In this case, we simply display the error message using $e-<getMessage().
Flowchart:
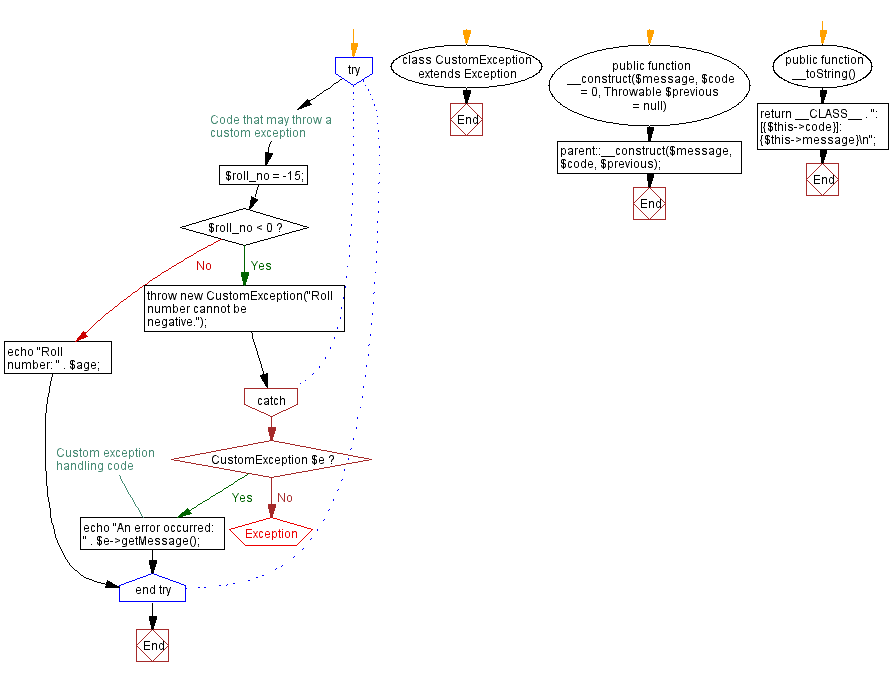
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Basic usage of try-catch blocks.
Next: Divide with zero denominator check.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics