PHP Exception Handling: Divide with zero denominator check
PHP Exception Handling: Exercise-3 with Solution
Write a PHP program that implements a PHP function that divides two numbers but throws an exception if the denominator is zero.
Sample Solution:
PHP Code :
<?php
function divideNumbers($numerator, $denominator) {
if ($denominator === 0) {
throw new Exception("Division by zero is not allowed.");
}
return $numerator / $denominator;
}
try {
$result = divideNumbers(200, 0);
echo "Result: " . $result;
} catch (Exception $e) {
echo "An error occurred: " . $e->getMessage();
}
?>
Sample Output:
An error occurred: Division by zero is not allowed.
Explanation:
In the above exercise,
- The divideNumbers() function takes two parameters, $numerator and $denominator. Inside the function, we check if the $denominator is zero. If it is, we throw a new instance of the Exception class with the message "Division by zero is not allowed."
- The function returns the division result if the denominator is non-zero.
- In the main code, we call the divideNumbers() function with arguments 10 and 0, which triggers an exception. The exception is caught in the catch block, and we display the error message using $e-<getMessage().
Flowchart:
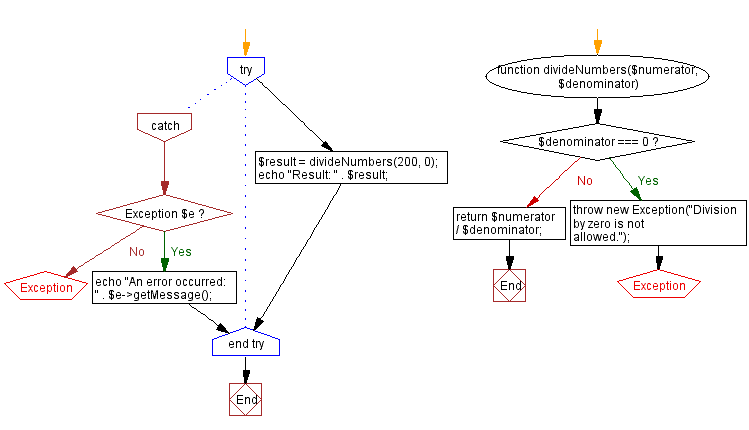
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Creating and throwing custom exceptions.
Next: Try-Catch blocks for error messages.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics