PHP File Handling: Custom exception for missing file
PHP Exception Handling: Exercise-5 with Solution
Write a PHP program that reads data from a file and throws a custom exception if the file does not exist.
Sample Solution:
PHP Code :
<?php
class FileNotExistException extends Exception {
public function __construct($message, $code = 0, Throwable $previous = null) {
parent::__construct($message, $code, $previous);
}
public function __toString() {
return __CLASS__ . ": [{$this->code}]: {$this->message}\n";
}
}
function readDataFromFile($filename) {
if (!file_exists($filename)) {
throw new FileNotExistException("File does not exist: $filename");
}
// Read data from the file and return it
$data = file_get_contents($filename);
return $data;
}
try {
$filename = "test.txt";
$data = readDataFromFile($filename);
echo "Data from $filename: " . $data;
} catch (FileNotExistException $e) {
echo "An error occurred: " . $e->getMessage();
}
?>
Sample Output:
An error occurred: File does not exist: test.txt
Explanation:
In the above exercise,
- First, we define a custom exception class FileNotExistException that extends the base Exception class. The custom exception class has a constructor that calls the parent constructor and overrides the __toString() method. This is to provide a custom string representation of the exception.
- Next, we have a function readDataFromFile() that takes a filename as a parameter. Inside the function, we check if the file exists using file_exists() function. If the file does not exist, we throw an instance of the FileNotExistException class with a custom error message indicating the filename.
- If the file exists, we read the data from it using file_get_contents() and return it.
- In the main code, we call the readDataFromFile() function with "test.txt". If the file exists, it reads the data and displays it. If the file does not exist, it catches the FileNotExistException and displays the error message using $e-<getMessage().
Flowchart:
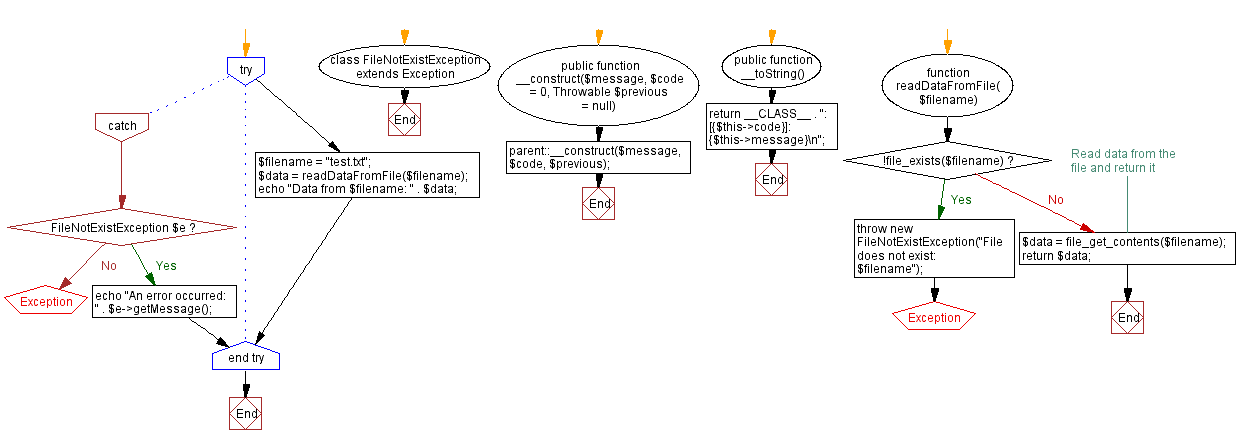
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Try-Catch blocks for error messages.
Next: Handling empty string exception.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics