PHP: Program to add a string to an existing file
PHP File Handling: Exercise-11 with Solution
Write a PHP program that appends a string to an existing file.
Sample Solution:
PHP Code :
<?php
$filename = "i:/test.txt";
$content = "This is the new content.";
try {
$fileHandle = fopen($filename, 'a');
if ($fileHandle === false) {
throw new Exception("Error opening the file for appending.");
}
fwrite($fileHandle, $content);
fclose($fileHandle);
echo "Content appended to the file successfully.";
} catch (Exception $e) {
echo "An error occurred: " . $e->getMessage();
}
?>
Sample Output:
Content appended to the file successfully.
Explanation:
In the above exercise -
- $filename variable holds the name of the file you want to append to, and a $content variable that holds the string to be appended to.
- Inside the try block, we use fopen() with the 'a' mode to open the file for appending. If there is an error opening the file, we throw an exception with the error message "Error opening the file for appending."
- We then use fwrite() to write the $content string to the file.
- After appending the content, we close the file using fclose().
- If everything succeeds, we display a success message indicating that content was appended to the file.
Flowchart:
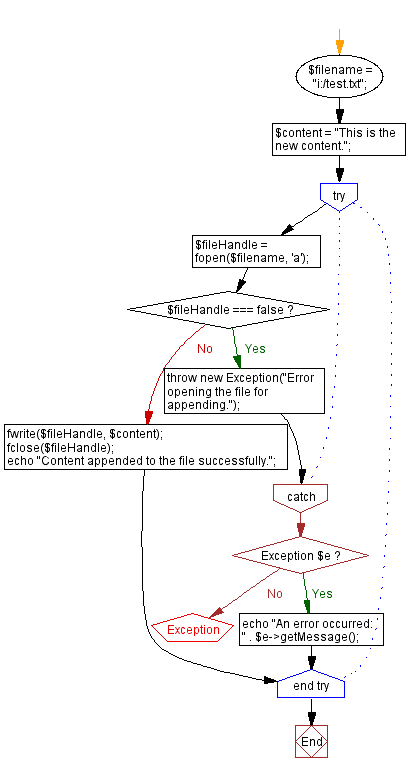
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Check if a file is writable or not.
Next: Display messages for different file types.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics