PHP function to check directory and create If not found
PHP File Handling: Exercise-13 with Solution
Write a PHP function to check if a directory exists and create it if it doesn't.
Sample Solution:
PHP Code :
<?php
function checkAndCreateDirectory($directory) {
try {
if (!is_dir($directory)) {
if (!mkdir($directory, 0777, true))
{
throw new Exception("Error creating the directory.");
}
echo "Directory created successfully.";
}
else
{
echo "Directory already exists.";
}
}
catch (Exception $e)
{
echo "An error occurred: " . $e->getMessage();
}
}
// Usage:
$directory = "i:/data1";
checkAndCreateDirectory($directory);
$directory = "i:/data2";
checkAndCreateDirectory($directory);
?>
Sample Output:
Directory already exists. Directory created successfully.
Explanation:
In the above exercise -
First, we define a function called checkAndCreateDirectory() that takes a directory path as input.
Inside the function, we use is_dir() to check if the directory already exists. If it doesn't exist, we use mkdir() to create the directory with 0777 permissions. The third argument "true" ensures that any necessary parent directories are also created. If creation succeeds, we display a success message. If there is an error creating the directory, we throw an exception with the error message "Error creating the directory."
If the directory already exists, we display the message "Directory already exists."
Flowchart:
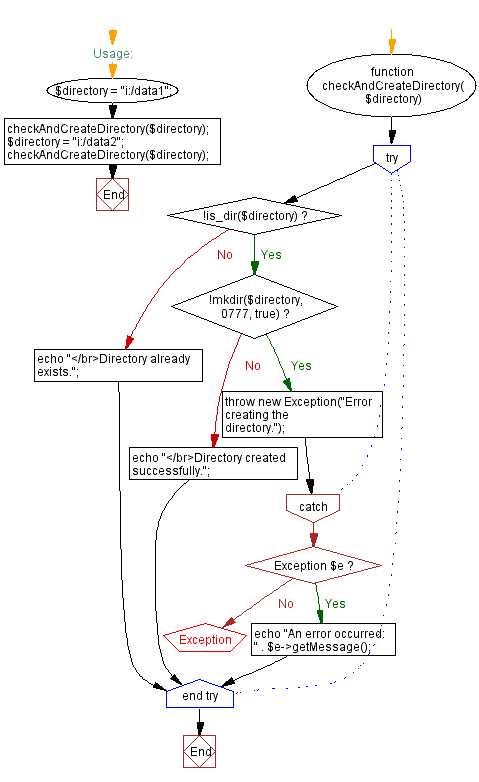
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Display messages for different file types.
Next: Locate and display line numbers.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics