PHP word search in text file: Locate and display line numbers
PHP File Handling: Exercise-14 with Solution
Write a PHP program that searches for a specific word in a text file and displays the line number(s) where it occurs.
Sample Solution:
PHP Code :
<?php
$filename = "i:/test.txt";
$searchWord = "PHP";
try {
$fileHandle = fopen($filename, 'r');
if ($fileHandle === false) {
throw new Exception("Error opening the file.");
}
$lineNumber = 1;
$found = false;
echo "Specific word: " . $searchWord;
while (($line = fgets($fileHandle)) !== false) {
if (stripos($line, $searchWord) !== false) {
echo "Word found on line " . $lineNumber . ": " . $line;
$found = true;
}
$lineNumber++;
}
if (!$found) {
echo "Word not found in the file.";
}
fclose($fileHandle);
} catch (Exception $e) {
echo "An error occurred: " . $e->getMessage();
}
?>
Sample Output:
Specific word: PHP Word found on line 1: PHP (recursive acronym for PHP: Hypertext Preprocessor) is a widely-used open source general-purpose scripting language that is especially suited for web development and can be embedded into HTML. Word found on line 3: The best way we learn anything is by practice and exercise questions. We have started this section for those (beginner to intermediate) who are familiar with PHP. Word found on line 5: Hope, these exercises help you to improve your PHP coding skills. Currently, following sections are available, we are working hard to add more exercises. Happy Coding!This is the new content.
Explanation:
In the above exercise -
- The $filename variable holds the name of the text file to search, and the $searchWord variable holds the specific word to search for.
- Inside the try block, we open the file using fopen() with the 'r' mode to read the file.
- Whenever there is an error opening a file, we throw an exception with the message "Error opening the file."
- We then enter a loop using fgets() to read each line from the file. Inside the loop, we use stripos() to perform a case-insensitive search for the specific word in each line of the file. If the word is found, we display the line number and line content.
- The $lineNumber variable starts at 1 and keeps track of the line number.
- In the event that the word was not found ($found is false), we display the message "Word not found in the file."
Flowchart:
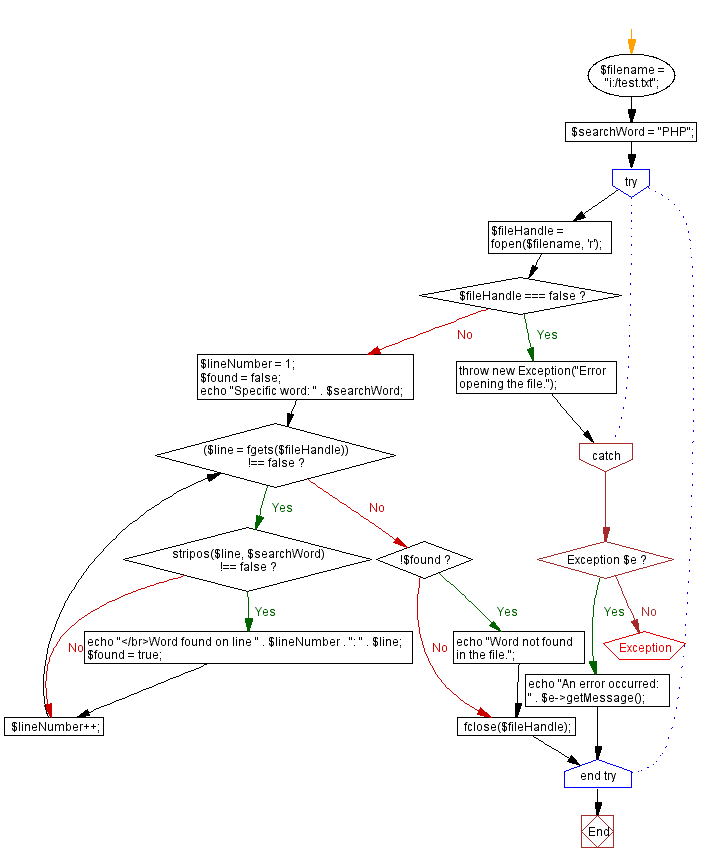
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: PHP function to check directory and create If not found.
Next: Read and display file contents.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics