PHP binary file: Read and display file contents
PHP File Handling: Exercise-15 with Solution
Write a PHP program that reads and displays the contents of a binary file.
Sample Solution:
PHP Code :
<?php
$filename = "i:/sample.bin";
try {
$fileHandle = fopen($filename, 'rb');
if ($fileHandle === false) {
throw new Exception("Error opening the file.");
}
$fileContents = fread($fileHandle, filesize($filename));
fclose($fileHandle);
echo "File Contents:\n";
echo $fileContents;
} catch (Exception $e) {
echo "An error occurred: " . $e->getMessage();
}
?>
Sample Output:
File Contents: SlickLWFNGWp1 �]� �]� �mBIN��]� (¬(z�%!PS-AdobeFont-1.0: Slick 1.0 %%Title: Slick %Version: 1.0 %%CreationDate: Sat Dec 7 02:08:58 2019 %%Creator: vagrant,,, % Generated by FontForge 20170924 (http://fontforge.sf.net/) %%EndComments 10 dict begin /FontType 1 def /FontMatrix [0.00195312 0 0 0.00195312 0 0 ]readonly def /FontName /Slick def /FontBBox {37 37 475 475 }readonly def /PaintType 0 def /FontInfo 8 dict dup begin /version (1.0) readonly def /FullName (slick) readonly def /FamilyName (Slick) readonly def /Weight (Book) readonly def /FSType 8 def /ItalicAngle 0 def /isFixedPitch false def /ascent 480 def end readonly def /Encoding StandardEncoding def currentdict end currentfile eexec t?��cl�Z���P��'0"��=o��S}���Z�Y����W� ��4*�S��w�(�skNSf�c���S^.a�H�]�+=�=O���_f��FW���-��~1�/�#"e� �0y�H闸"�!��7��O��9�b#C�䋒�ѤV�ܕ��P>ʳ�{Jr��]�F�$�w�������7ֵ�I�-��p�f{>�5���[��x�ۏiË���$r^BX�O�P֥ f��_L�(��z(��%�9�=� � `���Lʢ(O������.͊�d�eR{Np��x� <�(ۥ��DZ�`� ��a�S���� W�C֮�(C�@�-������}��#���_�Y��5�`:j pU2�>��,���7�Uٷ�������xO2*��wm�z�������i�Y@m��T� �E ��o[�3Z�k�wڕ����x� n~�d�;H�Ǒ�]+��������pa)?$.�T'¬�y��0�����Z� .��i:��}T7��3��� �&������G� �#L��t��k�~C�?m��쿮#��uƆ��&�d$����l��?�섮'���>Q|N'`(����7�*+hP¬��j��_X:���^�`[_�98��ڶ��5P�>�Oc\�^��fbm��W�8���98�'-{�Ђ�(\"ϗ�Y�#4�:�>��)���̪�.=�/R�OT�P!q#e�$F?�T��x� LQ4��6�L]c5�<�I8��gWUf��ͧ��H��o�<\�p�R�ȼ#�-y �v���`�H��jDz?#�J6Gݢ��?�'��W���7��4���wl��p1�ʜ�+���V�v��5P�L{.n�SX��;A�d�YUi��D��r��G ��a6��7�NQ���,��H����&���9Y���Qi���������Q����ZTVSI �L�/��p ����2�#�|���j �G`жfY��� M j�Fڑ�0000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000 0000000000000000000000000000000000000000000000000000000000000000 cleartomark (-(zzPOST ���������� ���������������¬"
Explanation:
In the above exercise -
- The $filename variable holds the name of the binary file you want to read and display.
- Inside the try block, we open the file using fopen() with the 'rb' mode to read the file in binary mode.
- If there is an error opening the file, we throw an exception with the error message "Error opening the file."
- We use fread() to read the file contents into a string variable called $fileContents. The filesize() function determines the size of the file.
- After reading the contents, we close the file using fclose().
- Finally, we display the file contents using the echo statement.
Flowchart:
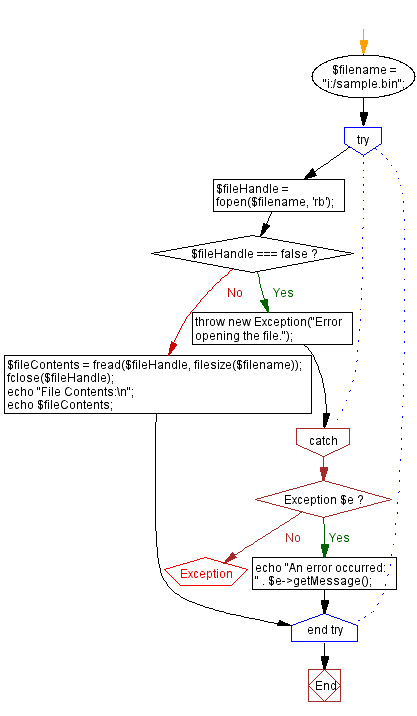
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Locate and display line numbers.
Next: Read and extract data from an XML file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics