PHP JSON file: Read and convert to an associative array
PHP File Handling: Exercise-17 with Solution
Write a PHP function that reads a JSON file and returns the decoded data as an associative array.
Sample json file (people.json)
{
"people" : [
{
"firstName": "Joe",
"lastName": "Jackson",
"gender": "male",
"age": 28,
"number": "7349282382"
},
{
"firstName": "James",
"lastName": "Smith",
"gender": "male",
"age": 32,
"number": "5678568567"
},
{
"firstName": "Emily",
"lastName": "Jones",
"gender": "female",
"age": 24,
"number": "456754675"
}
]
}
Sample Solution:
PHP Code :
<?php
function readJSONFile($filename) {
try {
$jsonContents = file_get_contents($filename);
if ($jsonContents === false) {
throw new Exception("Error reading the JSON file.");
}
$decodedData = json_decode($jsonContents, true);
if ($decodedData === null) {
throw new Exception("Error decoding the JSON data.");
}
return $decodedData;
} catch (Exception $e) {
echo "An error occurred: " . $e->getMessage();
}
}
// Usage:
$filename = "i:/people.json";
$data = readJSONFile($filename);
// Example usage of the returned associative array:
foreach ($data["people"] as $person) {
echo "Name: " . $person["firstName"] . " " . $person["lastName"] . "</br>";
echo "Age: " . $person["age"] . "</br>";
echo "Gender: " . $person["gender"] . "</br>";
echo "Number: " . $person["number"] . "</br>";
echo "------------------------</br>";
}
?>
Sample Output:
Name: Joe Jackson Age: 28 Gender: male Number: 7349282382 ------------------------ Name: James Smith Age: 32 Gender: male Number: 5678568567 ------------------------ Name: Emily Jones Age: 24 Gender: female Number: 456754675
Explanation:
In the above exercise -
- First, we use file_get_contents() to read the JSON file contents into a string variable named $jsonContents. If there is an error reading the file (e.g., the file doesn't exist or is not readable), we throw an exception with the message "Error reading the JSON file."
- We then use json_decode($jsonContents, true) to decode the JSON string into a PHP associative array. The second argument true specifies that we want an associative array rather than an object. If there is an error decoding the JSON data (e.g., the JSON format is invalid), we throw an exception with the message "Error decoding the JSON data."
- If the file is successfully read and the JSON data is decoded, we return the resulting associative array from the function.
- After the function definition, we have an example usage section where we call the readJSONFile($filename) function. In this example, we pass the filename "i:/people.json" to the function and store the returned associative array in a variable named $data.
- We then iterate over the "people" array within the $data associative array using a foreach loop. For each person, we access their properties (firstName, lastName, age, gender, and number) and display them using echo. We add HTML line breaks (</br>) to format the output.
Flowchart:
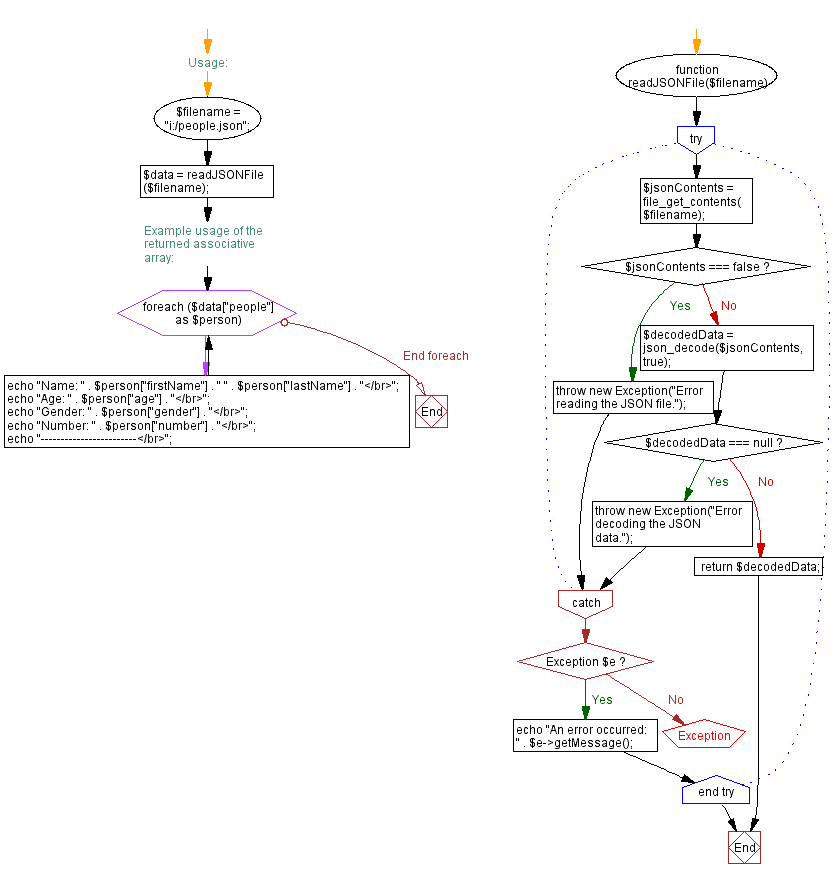
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Read and extract data from an XML file.
Next: PHP function to calculate the MD5 checksum of a file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics