PHP function to calculate the MD5 checksum of a file
PHP File Handling: Exercise-18 with Solution
Write a PHP function to calculate the MD5 checksum of a file.
Sample Solution:
PHP Code :
<?php
function calculateFileChecksum($filename) {
try {
if (!file_exists($filename)) {
throw new Exception("File does not exist.");
}
$checksum = md5_file($filename);
if ($checksum === false) {
throw new Exception("Error calculating MD5 checksum.");
}
return $checksum;
} catch (Exception $e) {
echo "An error occurred: " . $e->getMessage();
}
}
// Usage:
$filename = "i:/test.txt";
$checksum = calculateFileChecksum($filename);
echo "MD5 Checksum: " . $checksum . "\n";
?>
Sample Output:
MD5 Checksum: 4271622bc79aba1829f5612e0400a034
Explanation:
In the above exercise -
- The function calculateFileChecksum($filename) takes the filename as input.
- First, we check if the file exists using file_exists(). If the file does not exist, we throw an exception with the error message "File does not exist."
- We then use the md5_file() function to calculate the MD5 checksum of the file. This function reads the file contents and computes the MD5 hash value.
- If there is an error calculating the MD5 checksum, we throw an exception with the error message "Error calculating MD5 checksum."
- Finally, we return the computed MD5 checksum from the function.
Flowchart:
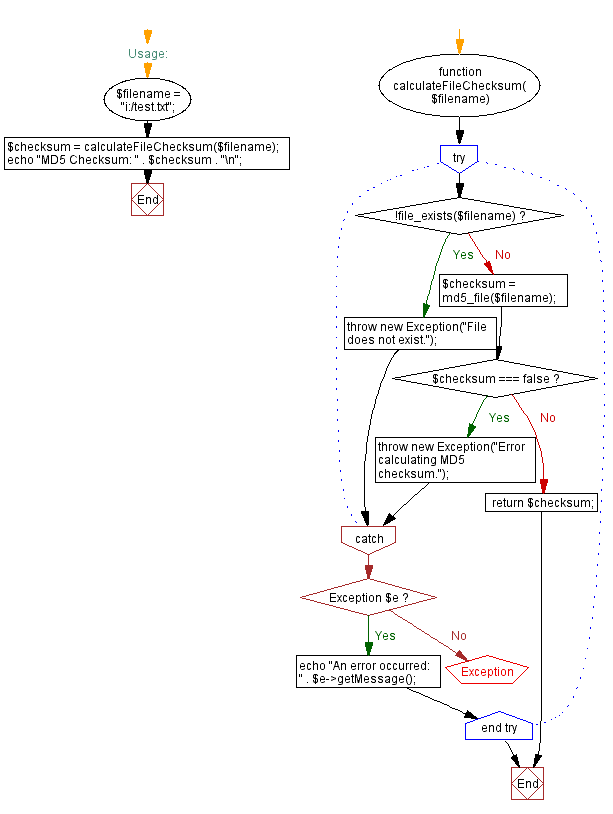
PHP Code Editor:
Contribute your code and comments through Disqus.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics