PHP program to read and display CSV data
PHP File Handling: Exercise-5 with Solution
Write a PHP program that reads a CSV file and displays the data in a tabular format.
Sample Solution:
PHP Code :
<?php
$filename = "i:/stock_data.csv";
try {
$fileHandle = fopen($filename, 'r');
if ($fileHandle === false) {
throw new Exception("Error opening the file.");
}
echo "";
while (($data = fgetcsv($fileHandle)) !== false) {
echo "";
foreach ($data as $cell) {
echo "" . htmlspecialchars($cell) . " ";
}
echo " ";
}
echo "
";
fclose($fileHandle);
} catch (Exception $e) {
echo "An error occurred: " . $e->getMessage();
}
?>
Sample Output:
Date Open High Low Close Adj Close Volume 01-04-2020 1122 1129.689941 1097.449951 1105.619995 1105.619995 2343100 02-04-2020 1098.26001 1126.859985 1096.400024 1120.839966 1120.839966 1964900 03-04-2020 1119.015015 1123.540039 1079.810059 1097.880005 1097.880005 2313400 06-04-2020 1138 1194.660034 1130.939941 1186.920044 1186.920044 2664700 07-04-2020 1221 1225 1182.22998 1186.51001 1186.51001 2387300 08-04-2020 1206.5 1219.069946 1188.160034 1210.280029 1210.280029 1975100 09-04-2020 1224.079956 1225.569946 1196.734985 1211.449951 1211.449951 2175400
Explanation:
In the above exercise -
- The $filename variable holds the name of the CSV file from which we want to read and display data.
- Inside the try block, we open the file using fopen() with the 'r' mode to read the file.
- If there is an error opening the file, we throw an exception with the error message "Error opening the file."
- We then enter a loop using fgetcsv() to read each line of the CSV file and convert it into an array of values.
- Inside the loop, we iterate over each value in the array using a foreach loop and display it as a table cell (<td>).
- After processing all the lines in the file, we close the file using fclose().
- To display the data in a tabular format, we wrap the table rows (<tr>) and table cells (<td>) within the appropriate HTML tags.
Flowchart:
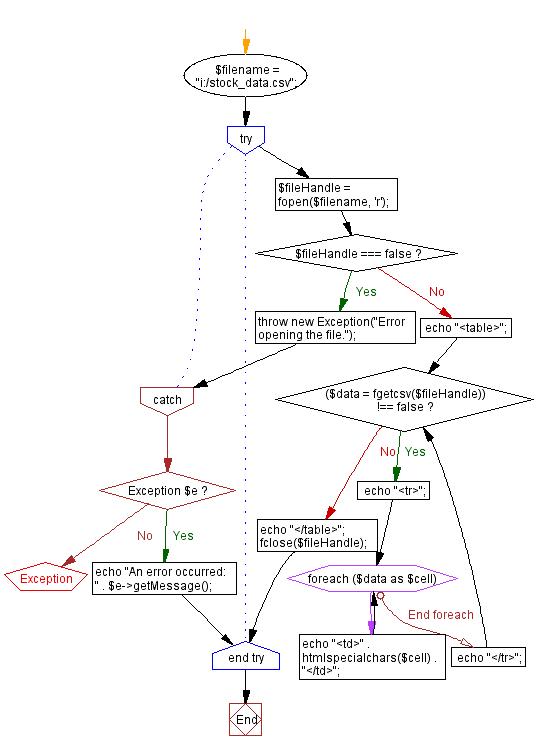
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: PHP function to write string to file.
Next: PHP script to rename a file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics