PHP function to copy a file
PHP File Handling: Exercise-7 with Solution
Write a PHP function that copies a file from one location to another.
Sample Solution:
PHP Code :
<?php
function copyFile($sourceFile, $destinationFile) {
try {
if (!file_exists($sourceFile)) {
throw new Exception("Source file does not exist.");
}
if (!copy($sourceFile, $destinationFile)) {
throw new Exception("Error copying the file.");
}
echo "File copied successfully.";
} catch (Exception $e) {
echo "An error occurred: " . $e->getMessage();
}
}
// Usage:
$sourceFile = "i:/test.txt";
$destinationFile = "i:/data/test.txt";
copyFile($sourceFile, $destinationFile);
?>
Sample Output:
File copied successfully.
Explanation:
In the above exercise -
- We define a function called copyFile() that takes two parameters: $sourceFile (the path of the source file) and $destinationFile (the path of the destination file).
- Inside the function, we use file_exists() to check if the source file exists. If it doesn't exist, we throw an exception with the error message "Source file does not exist."
- We then use the copy() function to copy the file from the source location to the destination location. If the copy operation succeeds, we display a success message. We throw an exception if the copy fails with the error message "Error copying the file."
Flowchart:
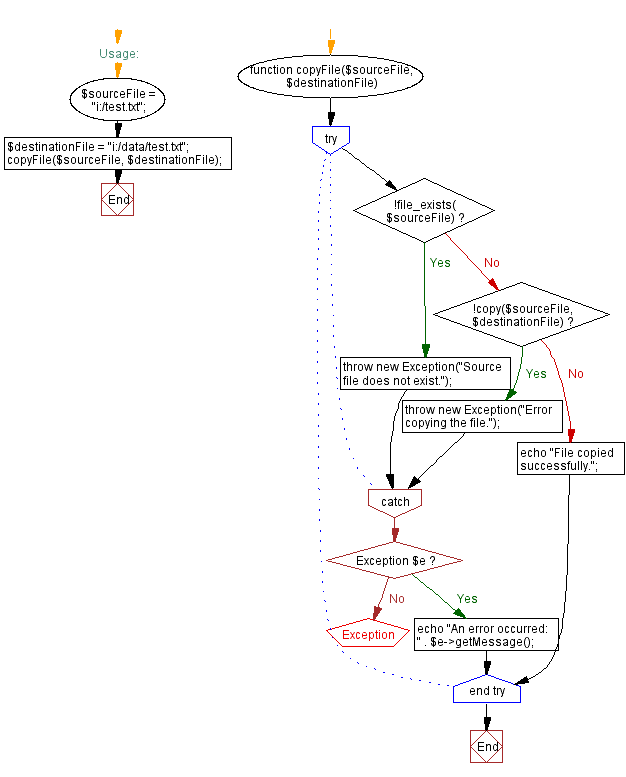
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: PHP script to rename a file.
Next: PHP program to delete a file.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics