PHP program to delete a file
PHP File Handling: Exercise-8 with Solution
Write a PHP program that deletes a specific file from the server.
Sample Solution:
PHP Code :
<?php
$filename = "i:/data/test.txt";
try {
if (file_exists($filename)) {
if (unlink($filename)) {
echo "File deleted successfully.";
} else {
throw new Exception("Error deleting the file.");
}
} else {
throw new Exception("File does not exist.");
}
} catch (Exception $e) {
echo "An error occurred: " . $e->getMessage();
}
?>
Sample Output:
File deleted successfully.
Explanation:
In the above exercise, we have a $filename variable that holds the name of the file we want to delete.
Inside the try block, we first check if the file exists using file_exists(). If it exists, we use the unlink() function to delete the file. If the deletion operation succeeds, we display a success message. If the deletion fails, we throw an exception with the error message "Error deleting the file."
If the file does not exist, we throw an exception with the error message "File does not exist."
In the catch block, we catch the exception and display an error message using $e->getMessage().
Flowchart:
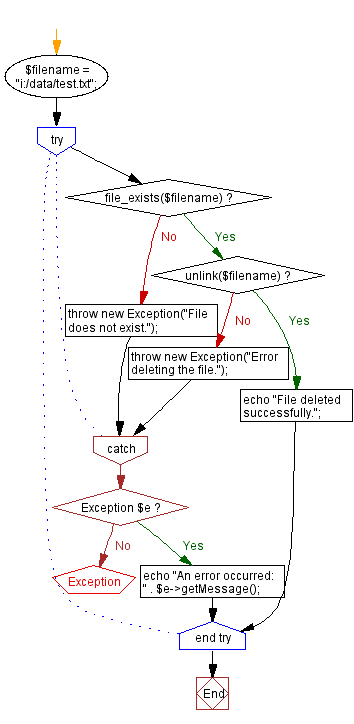
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: PHP function to copy a file.
Next: PHP function to check file size in human-readable format.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics