PHP function to check file size in human-readable format
PHP File Handling: Exercise-9 with Solution
Write a PHP function that checks the file size and displays it in human-readable format (KB, MB, GB).
Sample Solution:
PHP Code :
<?php
function formatFileSize($filename) {
$size = filesize($filename);
$units = array('B', 'KB', 'MB', 'GB', 'TB');
$formattedSize = $size;
for ($i = 0; $size >= 1024 && $i < count($units) - 1; $i++) {
$size /= 1024;
$formattedSize = round($size, 2);
}
return $formattedSize . ' ' . $units[$i];
}
// Usage:
$filename = 'i:/test.txt';
$fileSize = formatFileSize($filename);
echo 'File size: ' . $fileSize;
?>
Sample Output:
File size: 533 B
Explanation:
In the above exercise -
- We define a function called formatFileSize() that takes a filename as input.
- Inside the function, we use the filesize() function to get the file size in bytes.
- We define an array called $units that holds the unit labels for the file size (B, KB, MB, GB, etc.). The loop iterates through the units to determine the appropriate unit based on the file size.
- We divide the file size by 1024 in each iteration of the loop until the size is less than 1024 or we reach the last unit. We also update the formatted size to the rounded divided size value.
- The function returns the formatted size appended to the appropriate unit.
Flowchart:
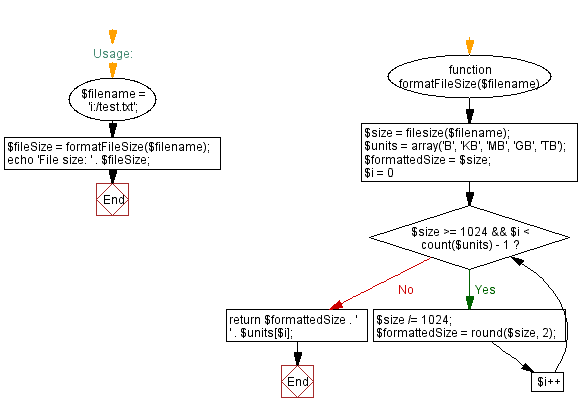
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: PHP program to delete a file.
Next: Check if a file is writable or not.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics