PHP calculator class: Perform Arithmetic operations
PHP OOP: Exercise-16 with Solution
Write a PHP class called 'Calculator' that has a private property called 'result'. Implement methods to perform basic arithmetic operations like addition and subtraction.
Sample Solution:
PHP Code :
<?php
class Calculator {
private $result;
public function __construct() {
$this->result = 0;
}
public function getResult() {
return $this->result;
}
public function add($number) {
$this->result += $number;
}
public function subtract($number) {
$this->result -= $number;
}
}
$calculator = new Calculator();
$calculator->add(4);
$calculator->add(5);
$calculator->subtract(3);
$result = $calculator->getResult();
echo "Result: " . $result;
?>
Sample Output:
Result: 6
Explanation:
In the above exercise -
- The "Calculator" class has a private property $result, which is initially set to 0 in the constructor method __construct().
- The getResult() method retrieves the current value of the $result property.
- The add($number) method takes a number as a parameter and adds it to the current value of $result.
- The subtract($number) method takes a number as a parameter and subtracts it from the current value of $result.
Flowchart:
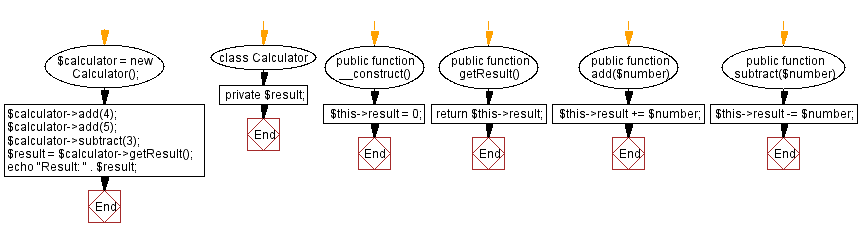
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: PHP class for file operations.
Next: Shopping cart class.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics