PHP Class: Singleton design pattern implementation
PHP OOP: Exercise-18 with Solution
Write a PHP class called 'Logger' that uses the singleton design pattern to ensure only one instance of the class can be created.
Sample Solution:
PHP Code :
<?php
class Logger {
private static $instance;
private $logs;
private function __construct() {
$this->logs = [];
}
public static function getInstance() {
if (self::$instance === null) {
self::$instance = new Logger();
}
return self::$instance;
}
public function log($message) {
$this->logs[] = $message;
}
public function getLogs() {
return $this->logs;
}
}
$logger = Logger::getInstance();
$logger->log("Log message 1</br>");
$logger->log("Log message 2</br>");
$logs = $logger->getLogs();
foreach ($logs as $log) {
echo $log . "\n";
}
?>
Sample Output:
Log message 1 Log message 2
Explanation:
In the above exercise -
- The "Logger" class has a private static property $instance to hold the singleton instance of the class.
- The constructor method __construct() is made private to prevent direct class instantiation.
- The static method getInstance() is implemented as a static factory method. It checks if an instance of the Logger class already exists. If not, it creates a new instance. This method ensures that only one instance of the class can be created.
- The log($message) method logs a message by adding it to the $logs array.
- The getLogs() method retrieves logs stored in the $logs array.
Flowchart:
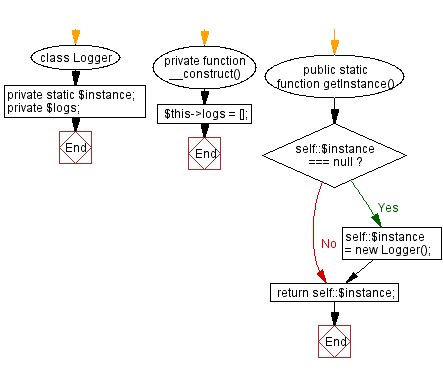
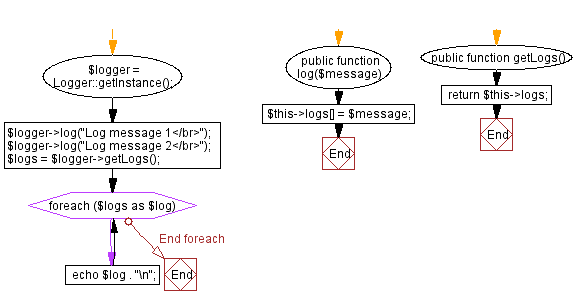
PHP Code Editor:
Contribute your code and comments through Disqus.
Previous: Shopping cart class.
Next: Email, password, and field validation.
What is the difficulty level of this exercise?
Test your Programming skills with w3resource's quiz.
- Weekly Trends and Language Statistics
- Weekly Trends and Language Statistics